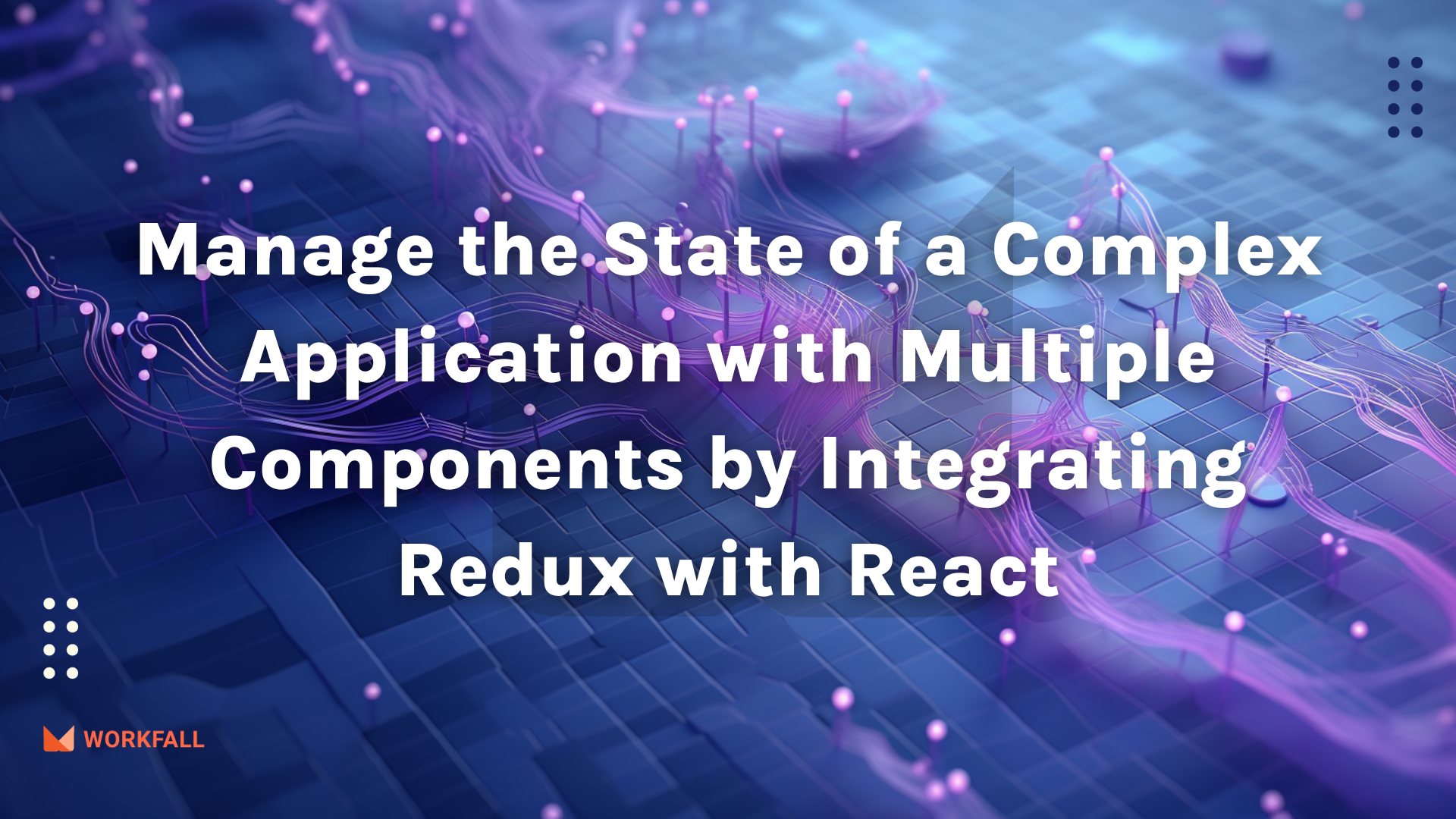
Navigating the complexities of state management is pivotal for controlling an application’s data, user interactions, and overall behavior. In this blog, we will explore step-by-step implementation of how to seamlessly manage state across multiple components by integrating Redux with React. Let’s start!
In this blog, we will cover:
- What is State Management?
- Are Your Favorite Websites and Apps Using State Management?
- What is Redux?
- Key Principles of Redux
- Hands-on
- Conclusion
What is State Management?
State management plays a pivotal role in the realm of application development, providing programmers with the means to regulate the app’s response to diverse events and user interactions.
This capability is instrumental in crafting dynamic and interactive interfaces, thereby enhancing the overall user experience.
The significance of state management becomes apparent in its ability to facilitate the creation of responsive applications that can adapt to changing conditions and user inputs.
It enables developers to efficiently handle data and control the behavior of components, ensuring a seamless and user-friendly application flow.
Are Your Favorite Websites and Apps Using State Management?
Numerous websites and applications, ranging from straightforward to intricate, leverage state management to optimize their functionality.
Widely adopted frameworks such as React, along with others like Angular.js, Vue.js, and Next.js, incorporate state management techniques to proficiently manage data and govern the behavior of various components within the application.
What is Redux?
Redux, a JavaScript library available as open-source, is designed to handle the state management of web applications. It is commonly used with React, but it can be employed with other JavaScript frameworks or libraries as well. Redux provides a predictable state container, meaning that the state of an application is stored in a single JavaScript object called the “store.”
The state within the store can only be modified by dispatching actions, which are plain JavaScript objects describing the change. Reducers serve as functions outlining how the state responds to an action, dictating its changes.They take the current state and an action as arguments, and they return to a new state.
Key Principles of Redux
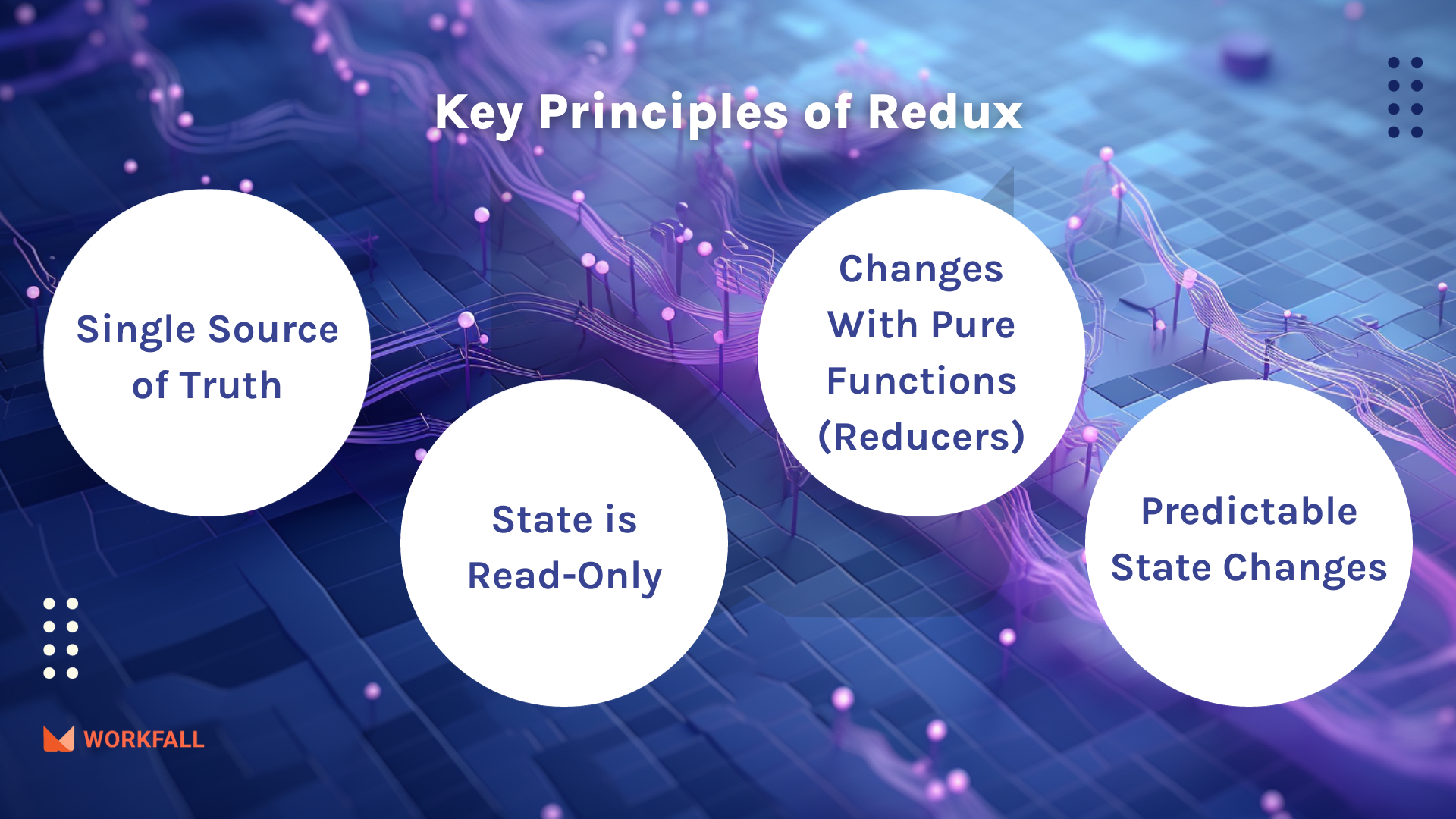
- Single Source of Truth: The entire state of the application is stored in a single store.
- State is Read-Only: The state can only be modified by dispatching actions.
- Changes are Made with Pure Functions (Reducers): Reducers take the current state and an action and return a new state.
- Predictable State Changes: With a single, predictable flow of data, it becomes easier to understand and debug the application.
Redux is particularly useful in managing complex state logic in large applications. It enables developers to maintain a clear and centralized understanding of the application’s state, making it easier to manage and reason about the flow of data.
Hands-on
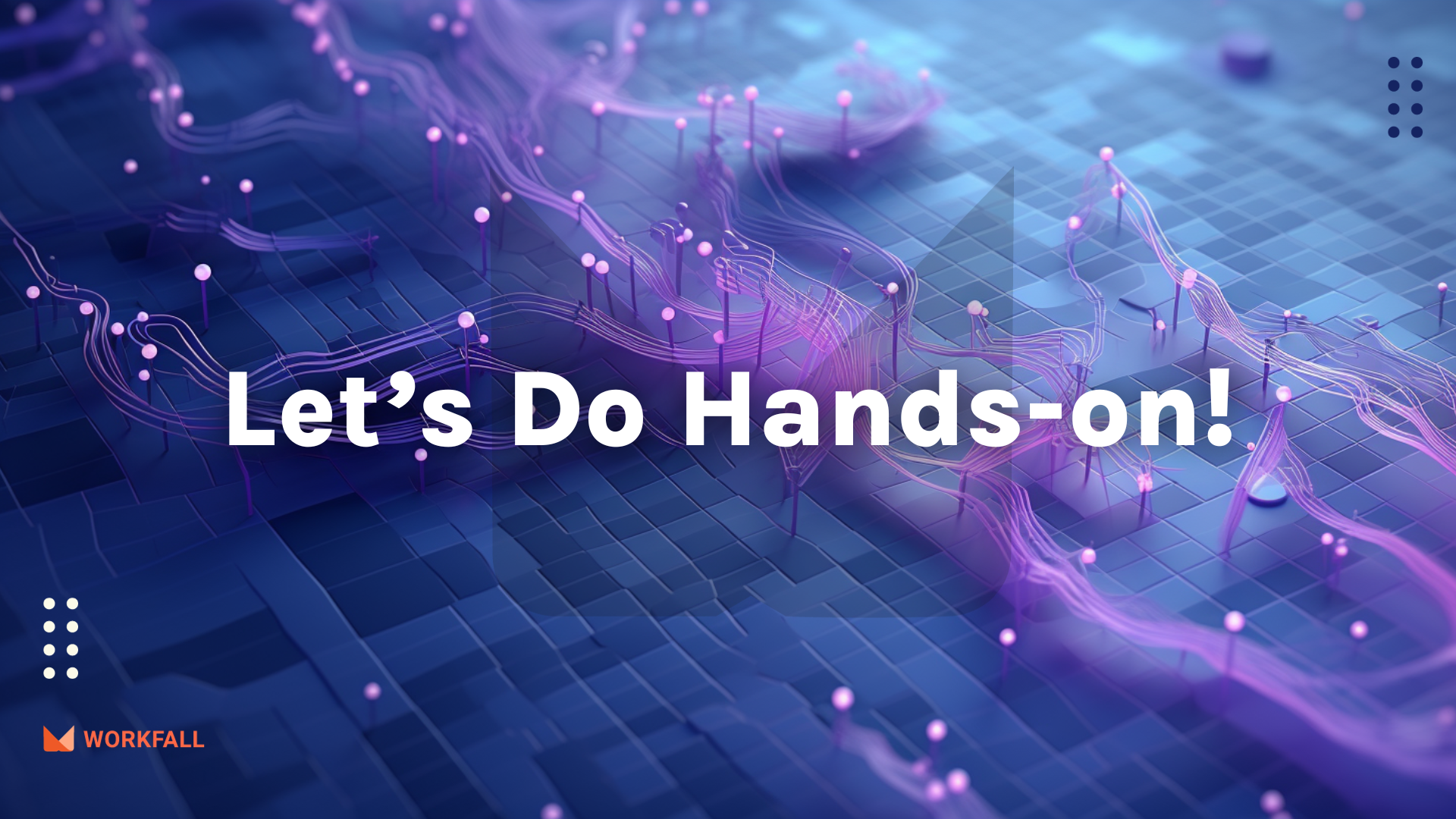
Required installations:
To perform the demo, you require the following installations:
react-redux: React-Redux is the official library that seamlessly integrates the React framework with the Redux state management library for efficient handling of state in React applications.
In this hands-on, we’re going to explore the process of creating a React application with Redux for optimal state management.
The journey begins by initiating a new directory on your local machine and setting up a React app in Visual Studio Code using the command “npx create-react-app blog-app.”
Following the app initialization, essential components such as ListPosts, AddPost, and PostDetails will be created. We’ll establish a Redux store by installing necessary libraries with the command and configure the store, reducers, and actions accordingly.
Navigating into the UI development, we’ll implement the AddPost component with fields like title, author_name, category, and content.
Adjustments to the App.js file, introduction of Bootstrap CDN in the index.html file, and styling of the application using provided CSS code in App.css will be part of the process.
The finalization of the application involves coding the PostDetails and ListPost components and integrating them into the application. We will perform thorough testing by adding new posts, viewing details, and seamlessly navigating a dynamically updated post list. By the conclusion of this blog, you will have gained valuable insights into building versatile React applications with Redux, showcasing effective state management and dynamic user interfaces.
Create a new directory on your local machine.
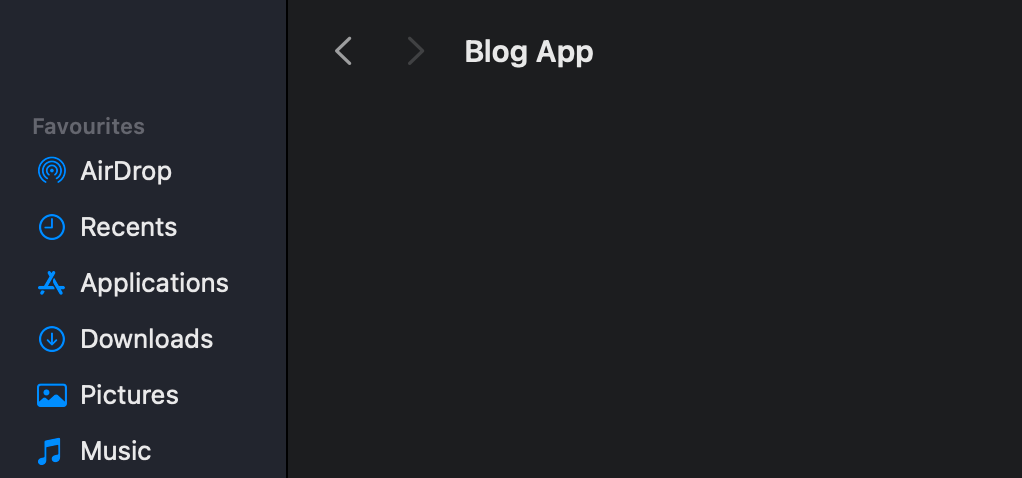
Open VS Code and click on open, to open the directory you just created.
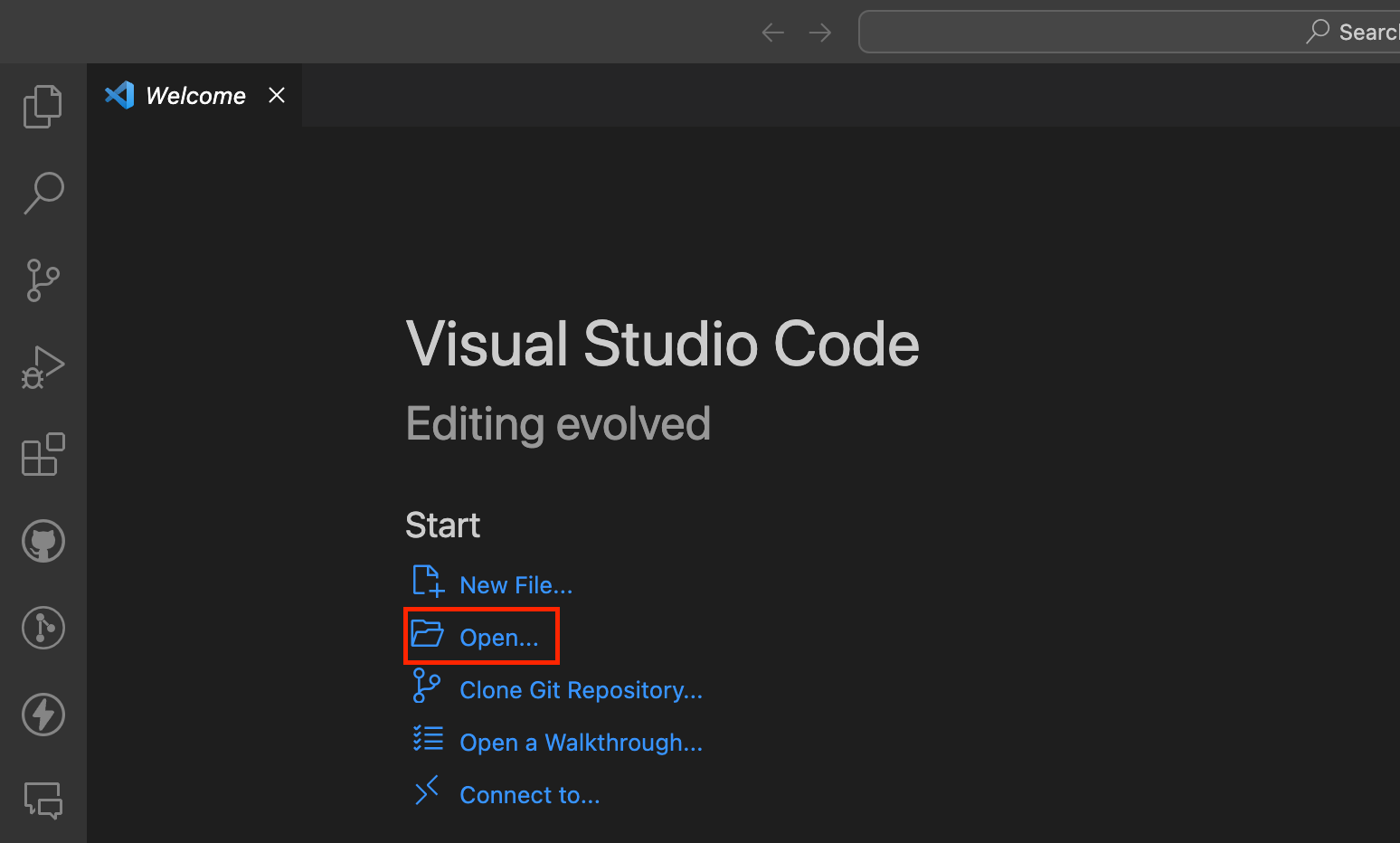
After opening the newly created directory, you will see a welcome screen like this below image.
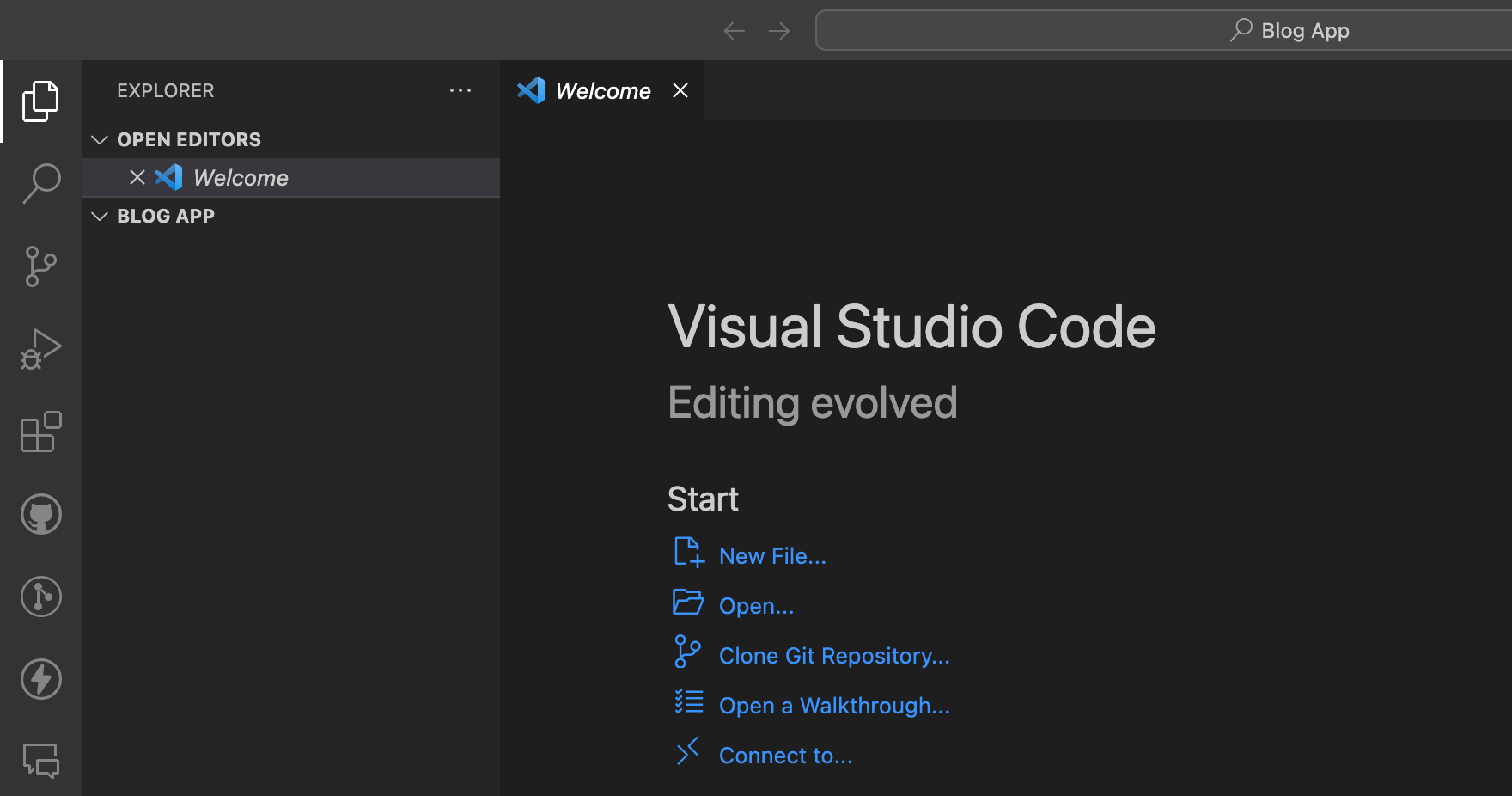
Open the VS Code Terminal and run the command “npx create-react-app blog-app”.

After executing the command, you will see the screen as shown in the image below.
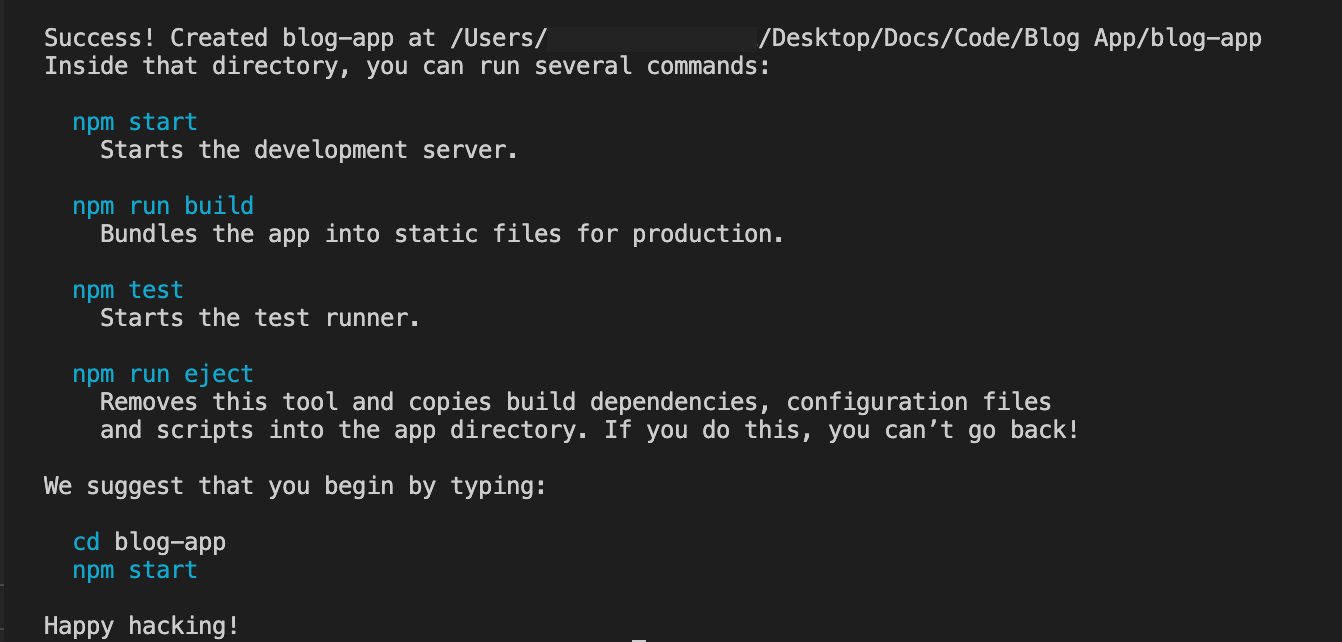
The next step is to change the active directory using the command shown in the image below.

Next, to see the app in action run the “npm start” command from the “blog-app” folder.

You will see the screen as shown in the image below.
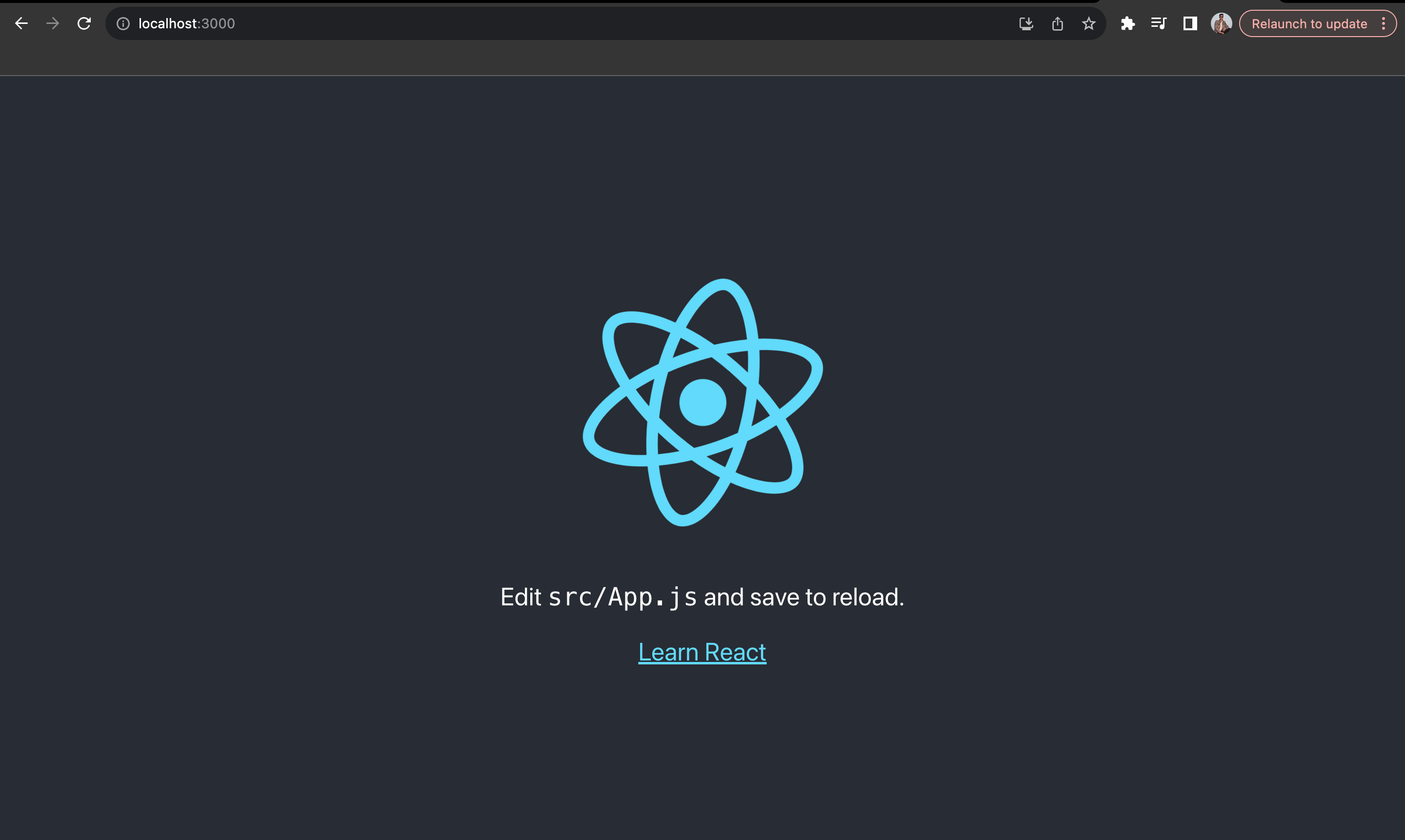
Now, we will make 3 different components namely ListPosts, AddPost, PostDetails.
For that, inside the “src” folder make a new folder and name it as components as shown in the image below.
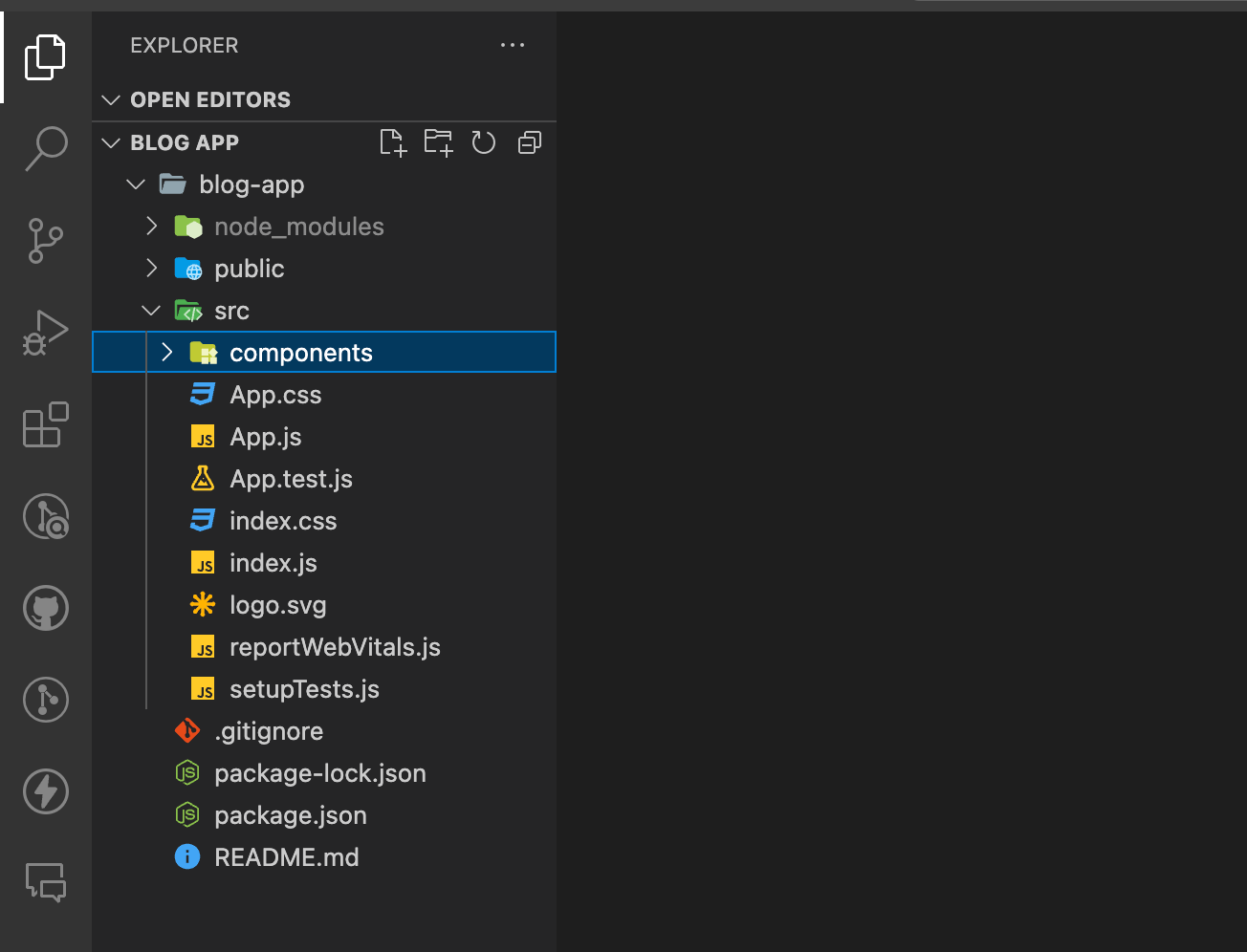
Inside the components folder, create three new files and name them as shown in the image below.
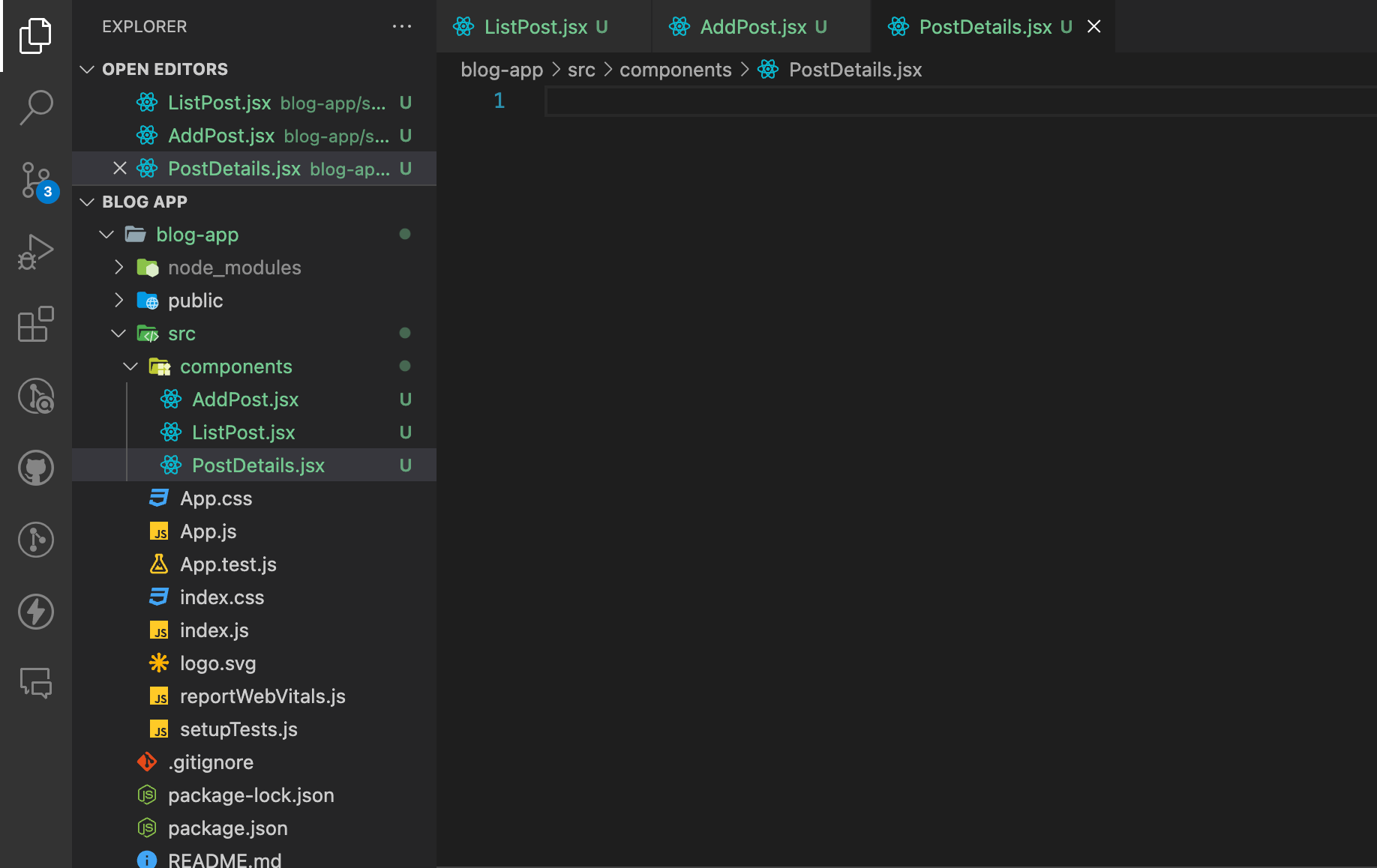
Create another folder inside “src” and name it as “store” as shown in the image below.
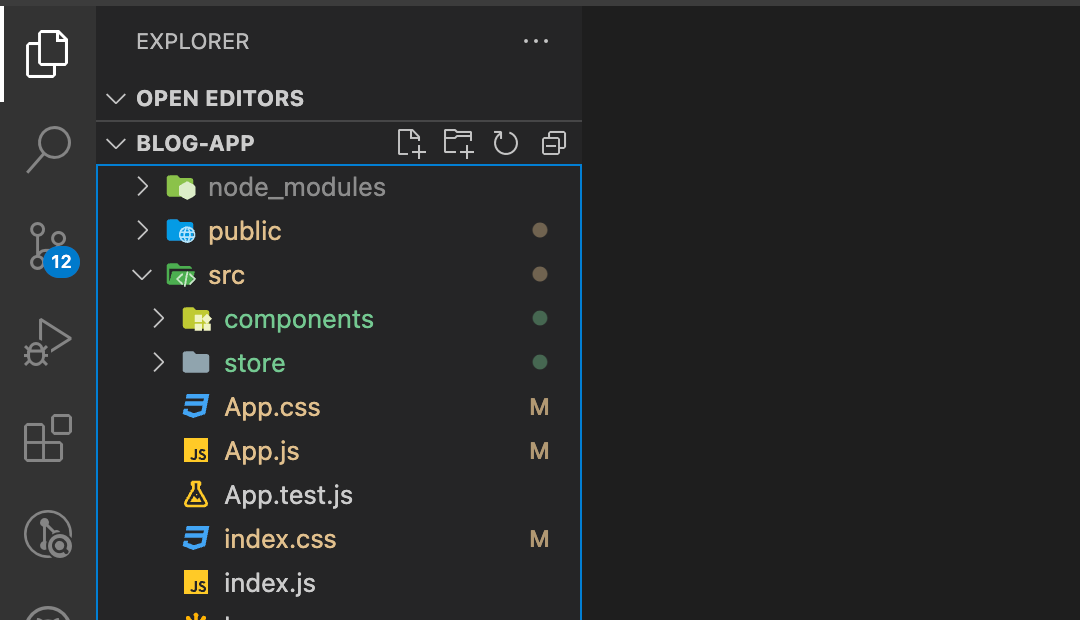
Create a new file inside the newly created folder and name it as “index.js” as shown in the image below.
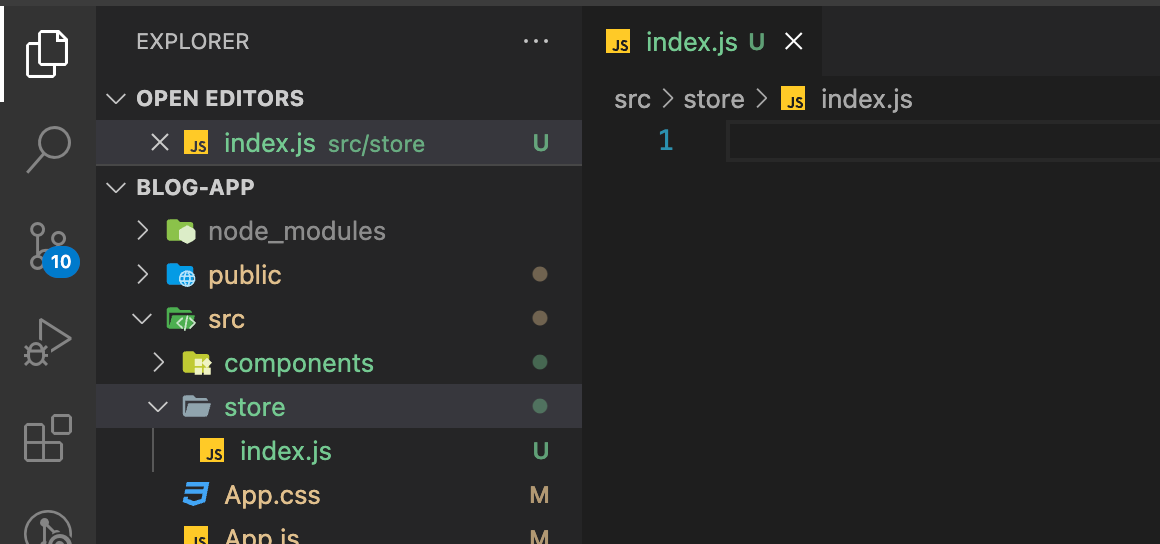
Now, we need to first install the redux library to be able to use it in our code.
For that, run the following command in the terminal “npm install redux react-redux”.

After executing the command, you will see the screen as shown in the image below.

Write the following code inside the “index.js” file in the “store” folder to create a Redux store using the createStore function from the Redux library. It uses the rootReducer to manage the state which we will create in the next step.
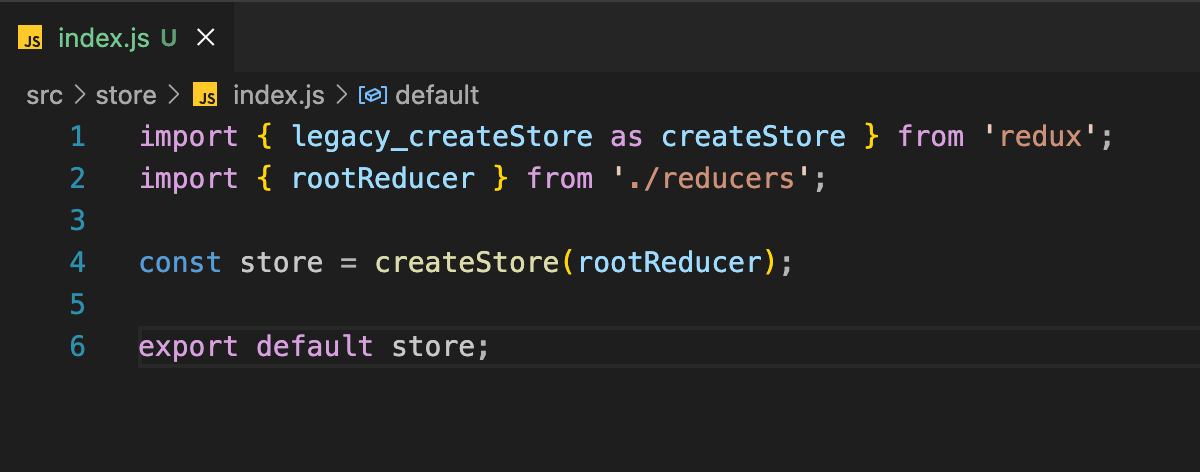
Create another file inside the “store” folder and name it as “reducers.js” as shown in the image below
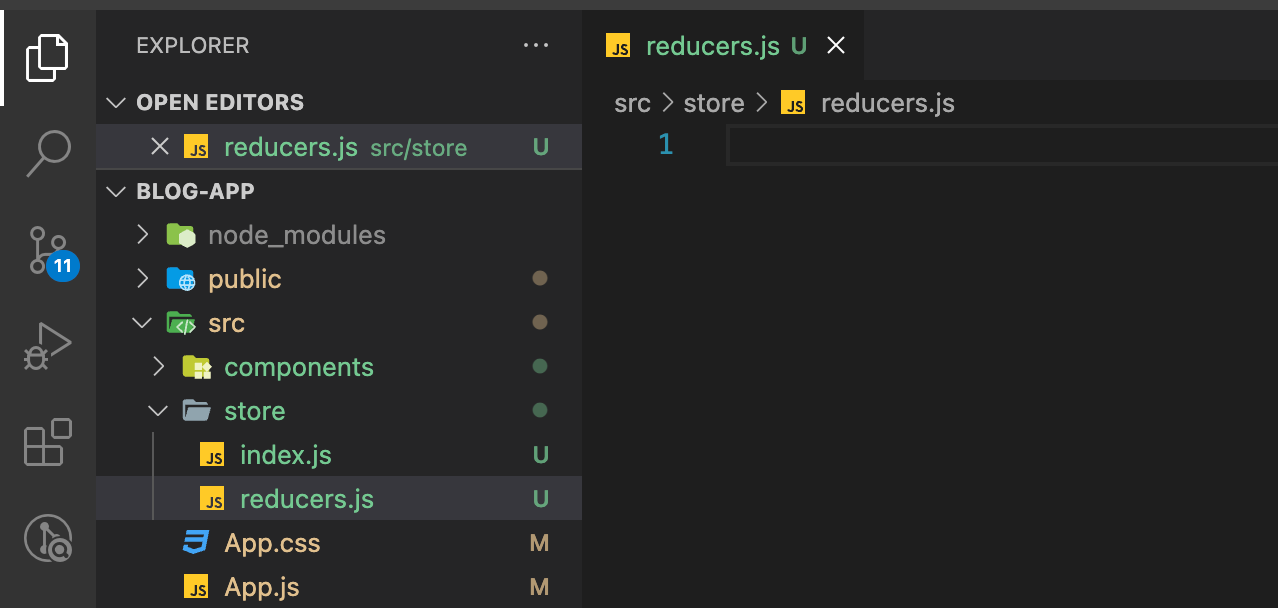
Write the following code inside the “reducers.js” file which will specify how the state will change in response to different actions. It initializes the state with an array of posts and handles the ‘ADD_POST’ action by adding a new post to the state.
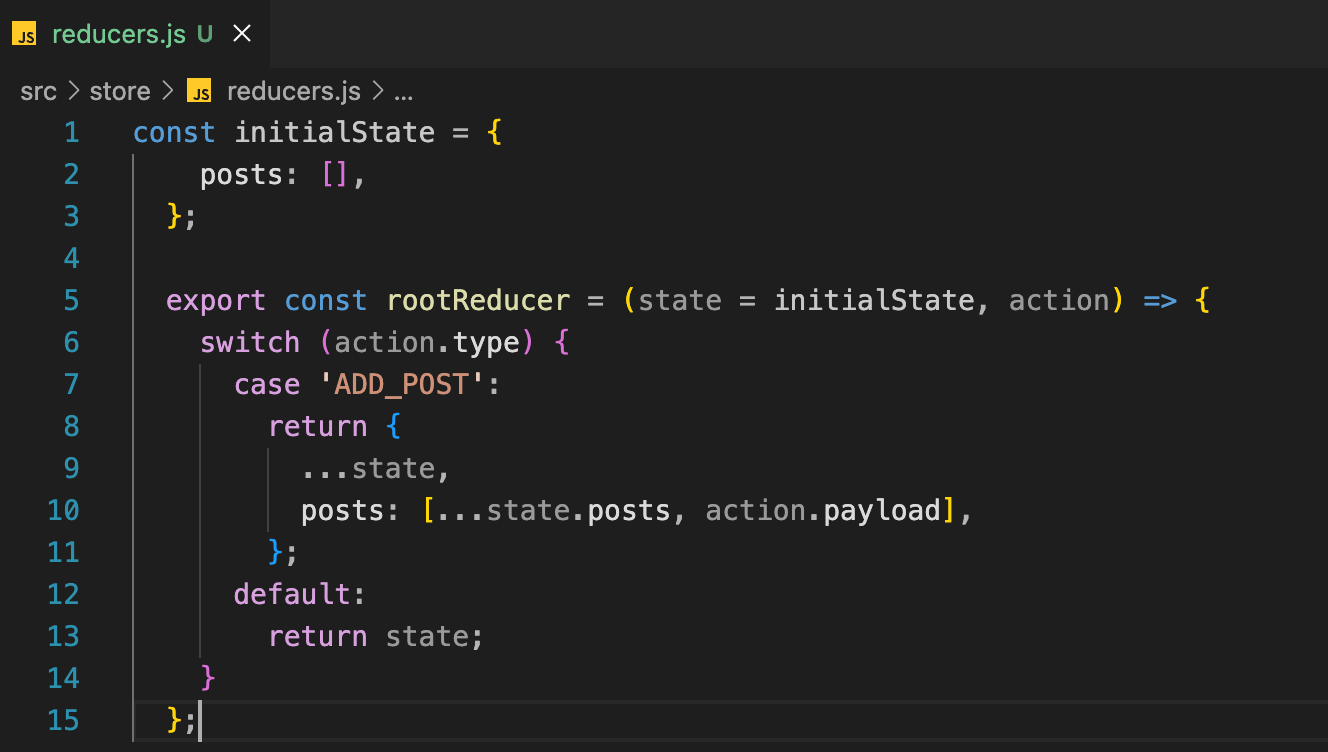
Create another file in the “store” folder and name it as “actions.js” as shown in the image below
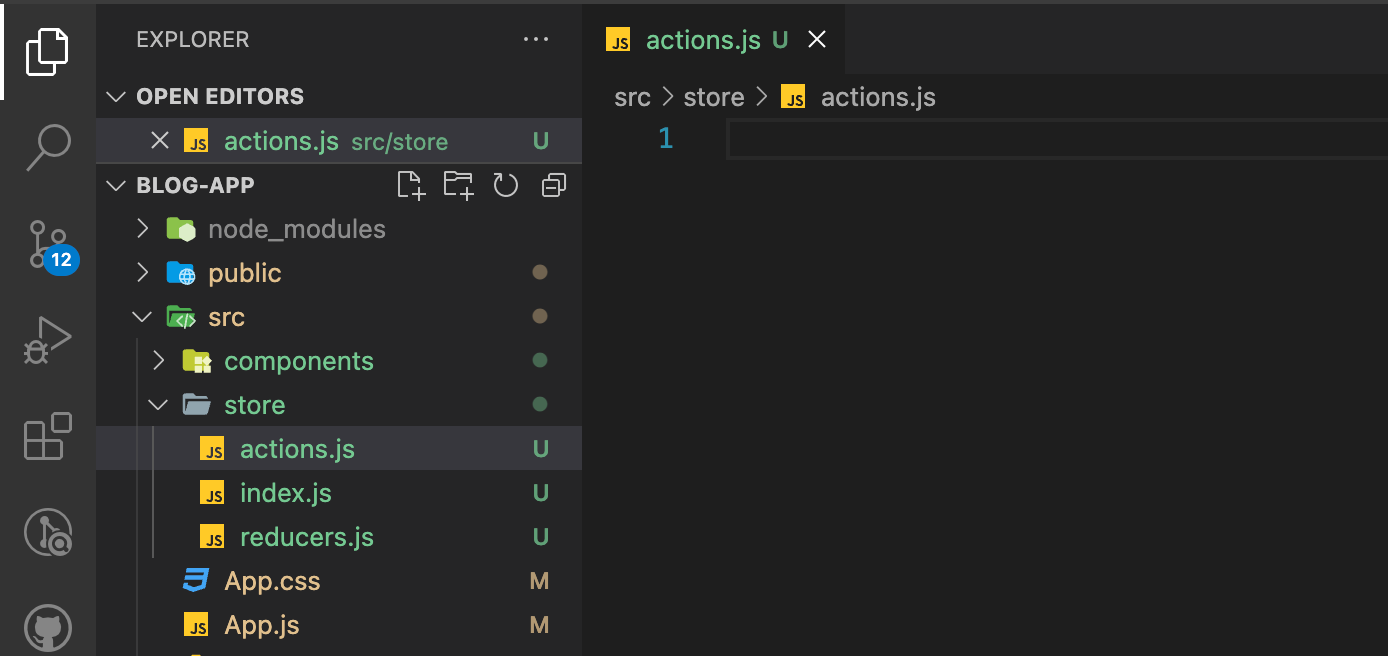
Write the following code inside the “actions.js” file which defines an action creator, addPost, which creates an action to add a post. Actions in Redux are plain JavaScript objects with a type field that describes the action and a payload field containing any additional data.
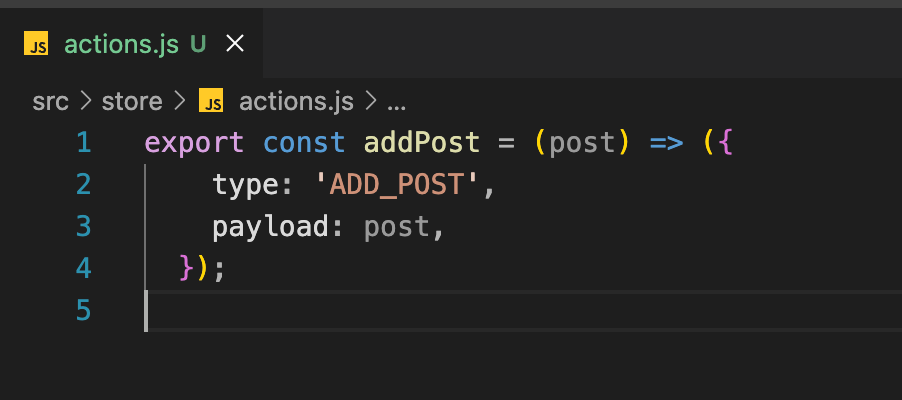
Now that we have created a store, reducer and defined action, it’s time to jump to the UI code that will use these redux functions.
First, let’s write the code for the “AddPost” component. Our post will have 4 fields such as “title”, “author_name”, “category” and, “content”. The final code for the “AddPost” component is given in the image below.
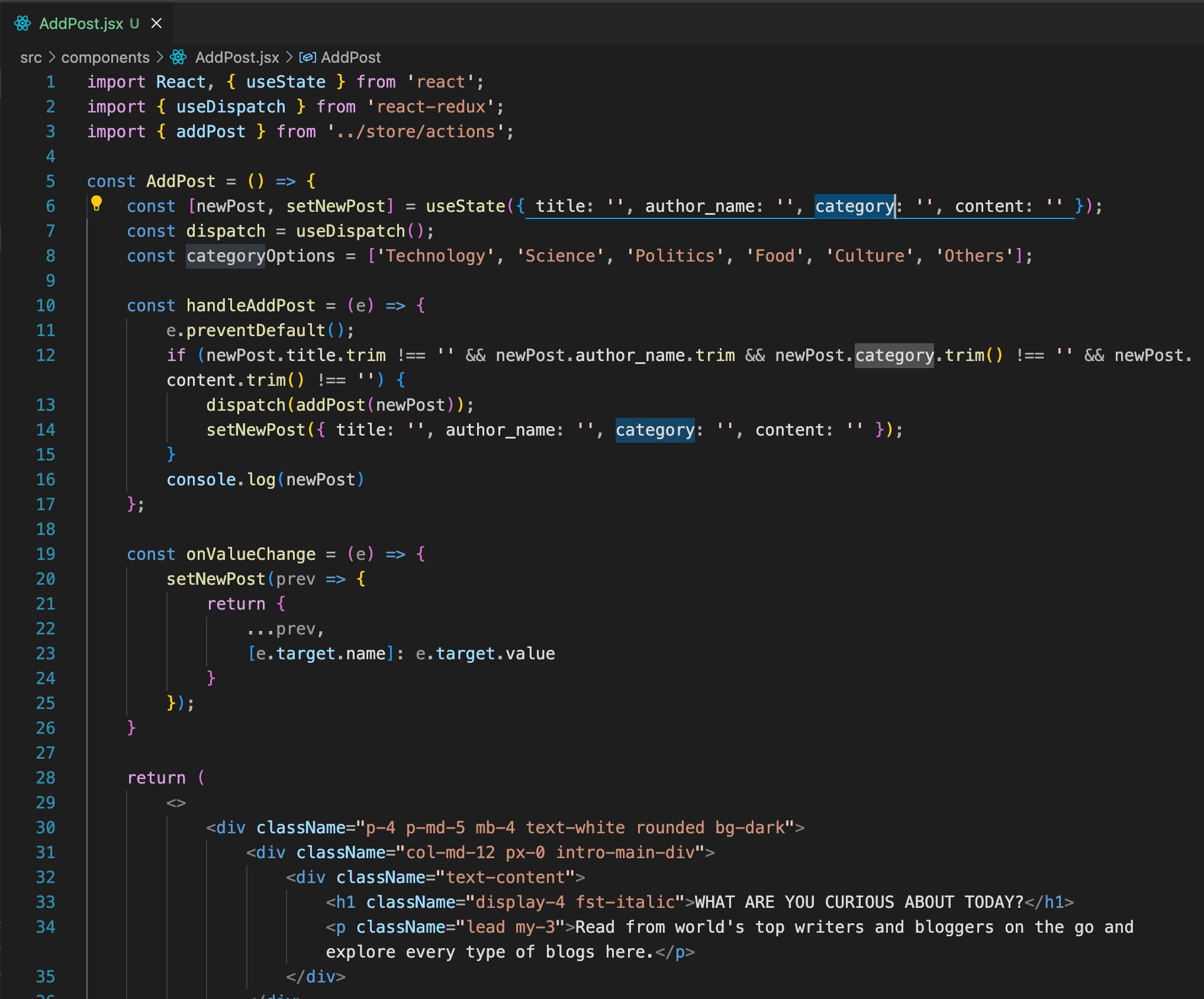
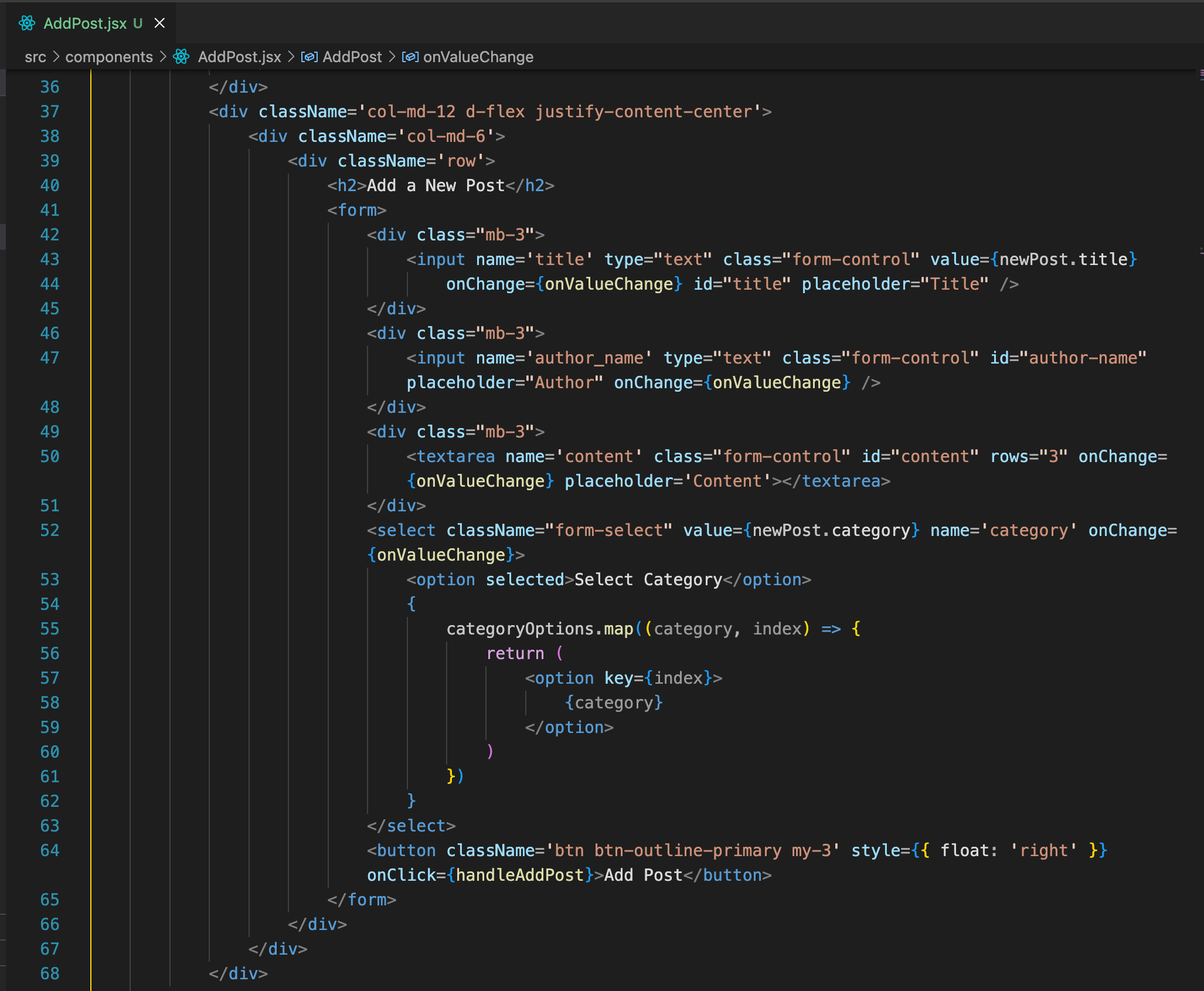
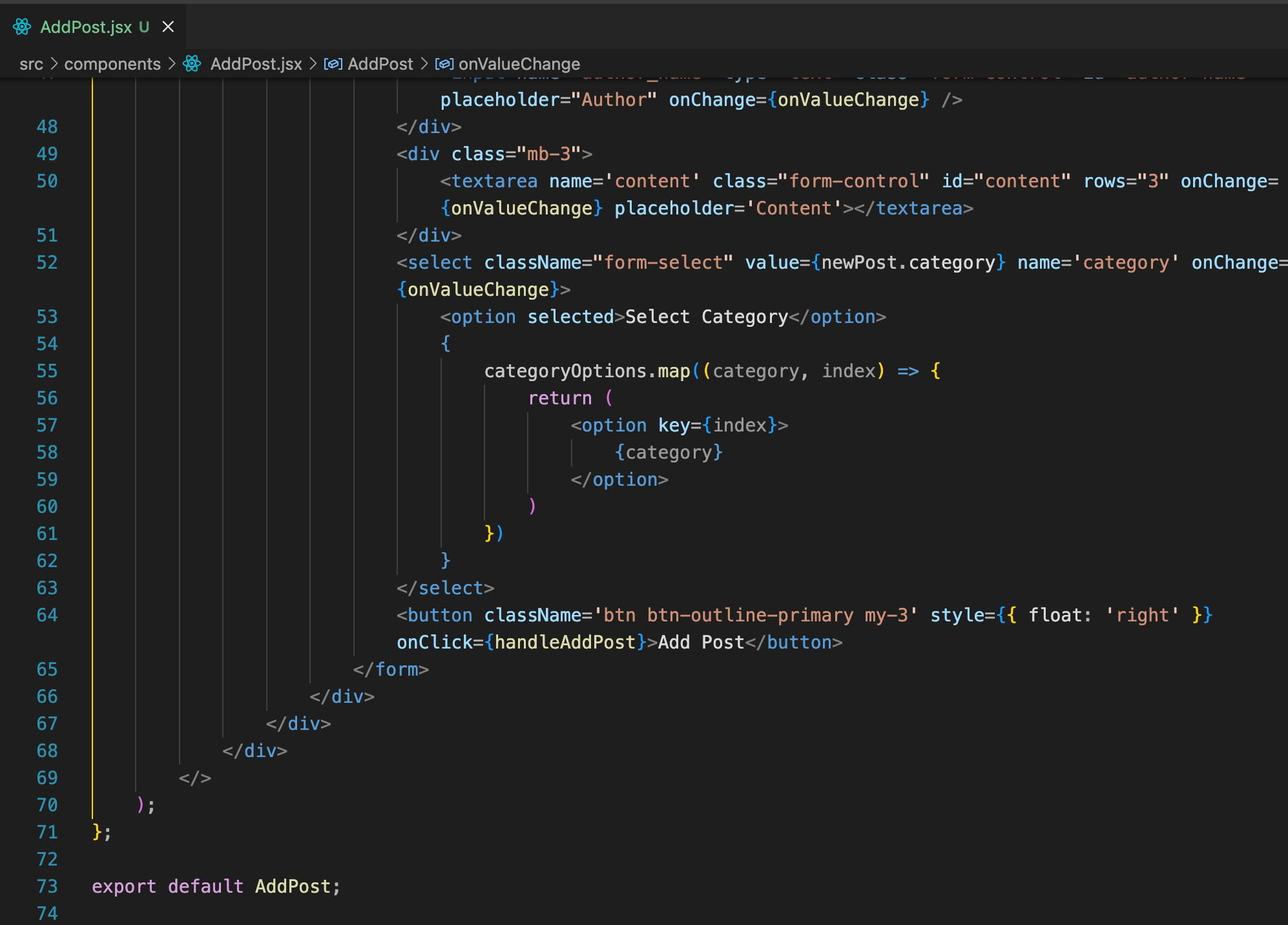
Now, let’s head toward the “App.js” file and add the following code as shown in the image below.
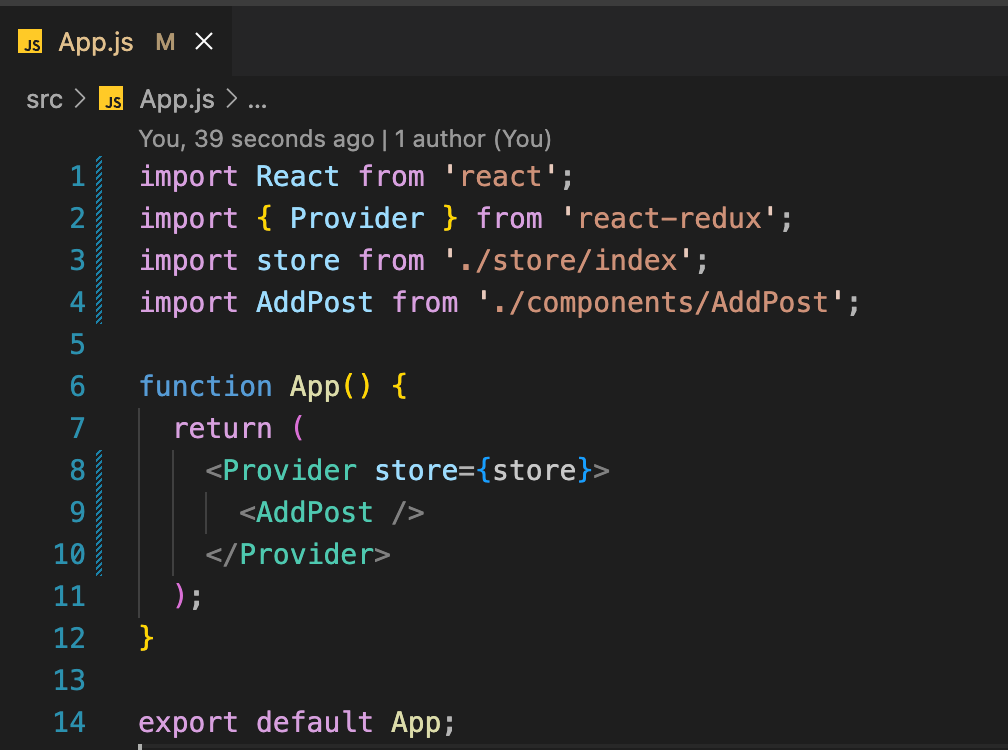
Now, we have to add the bootstrap cdn in our app.
For that, open the “index.html” file and paste the bootstrap cdn inside <head> tag as shown in the image below.
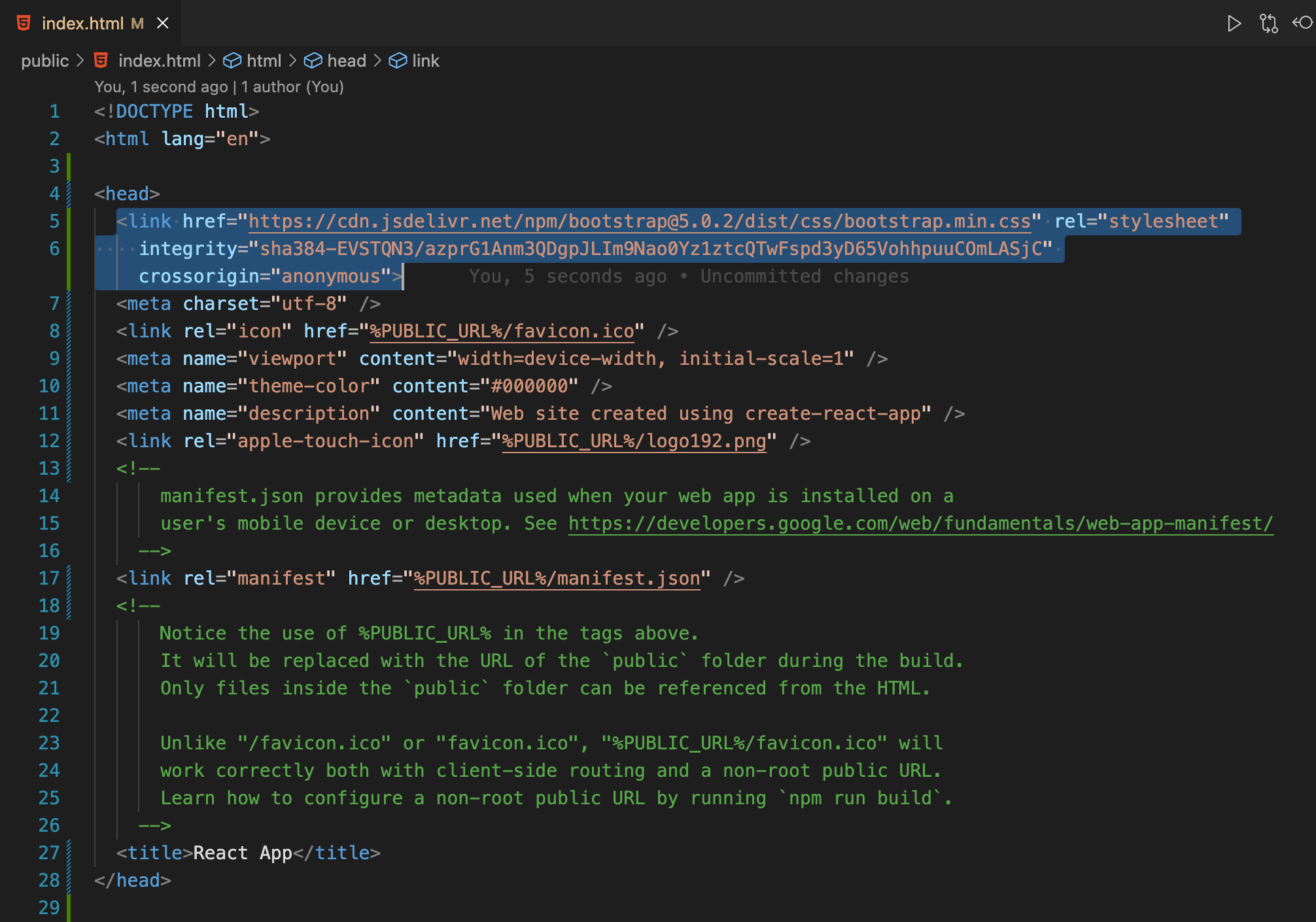
Write the following css code inside the “App.css” file to style our application.
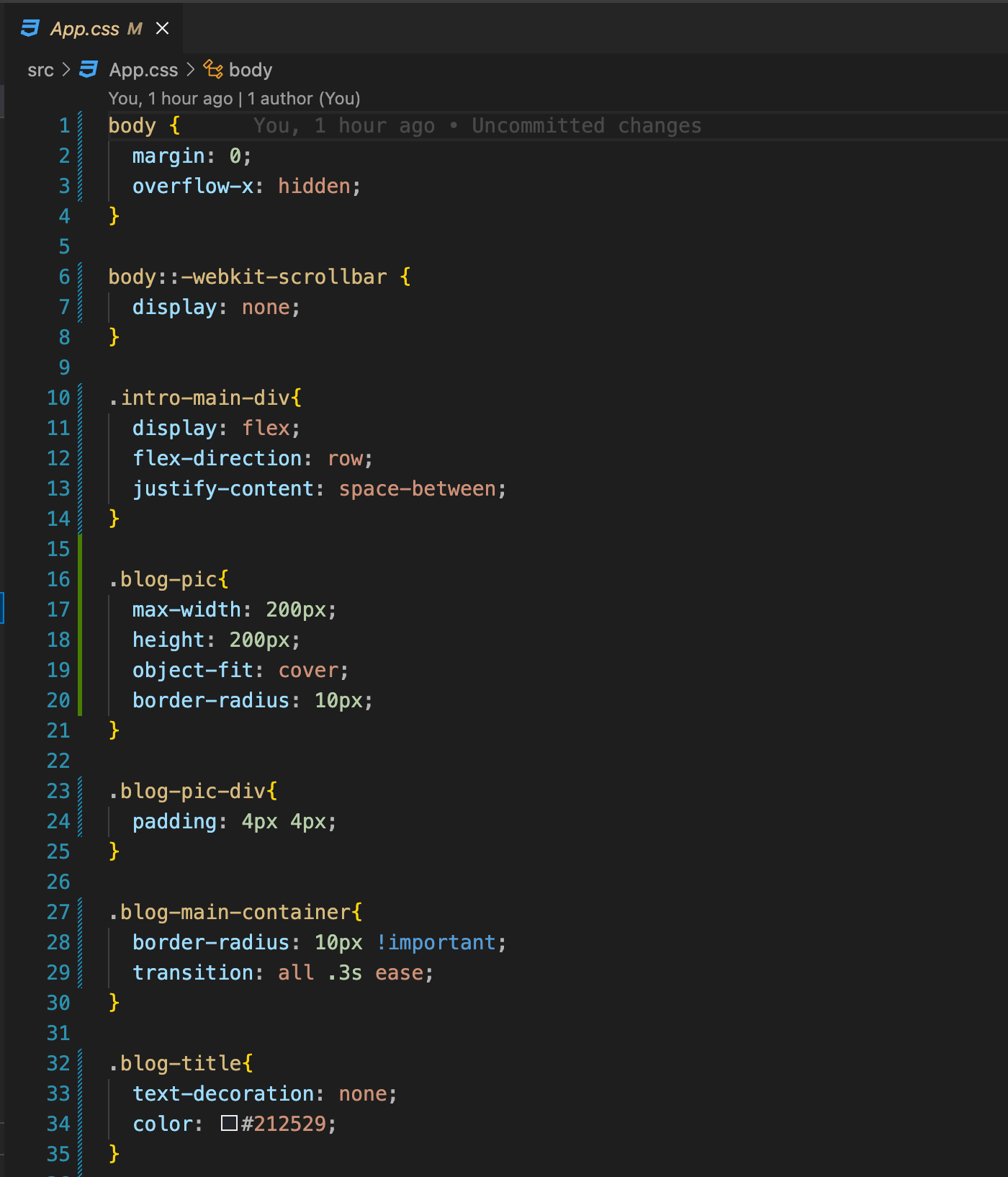
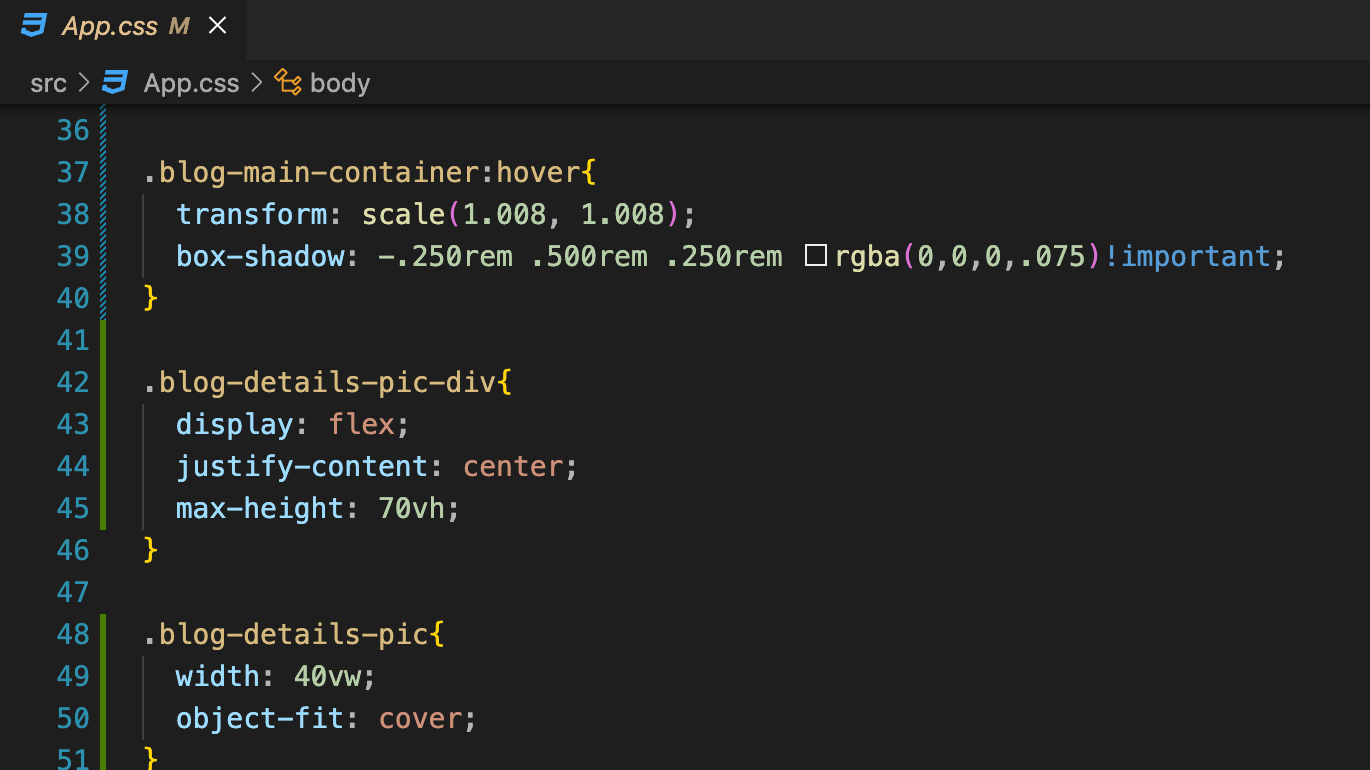
Open the browser and you will be able to see the screen as shown in the image below.
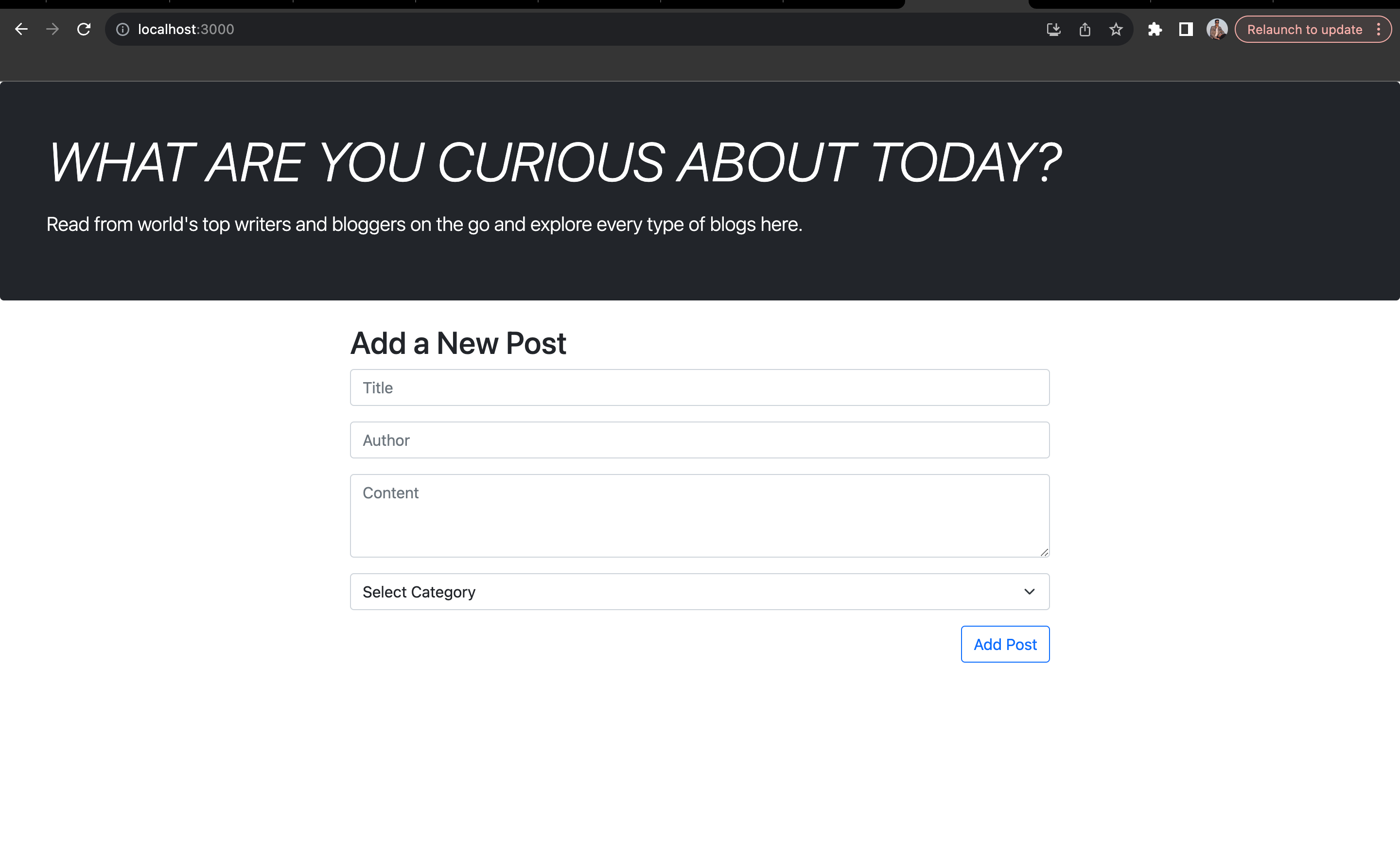
Now, let’s write some code for “PostDetails” component.
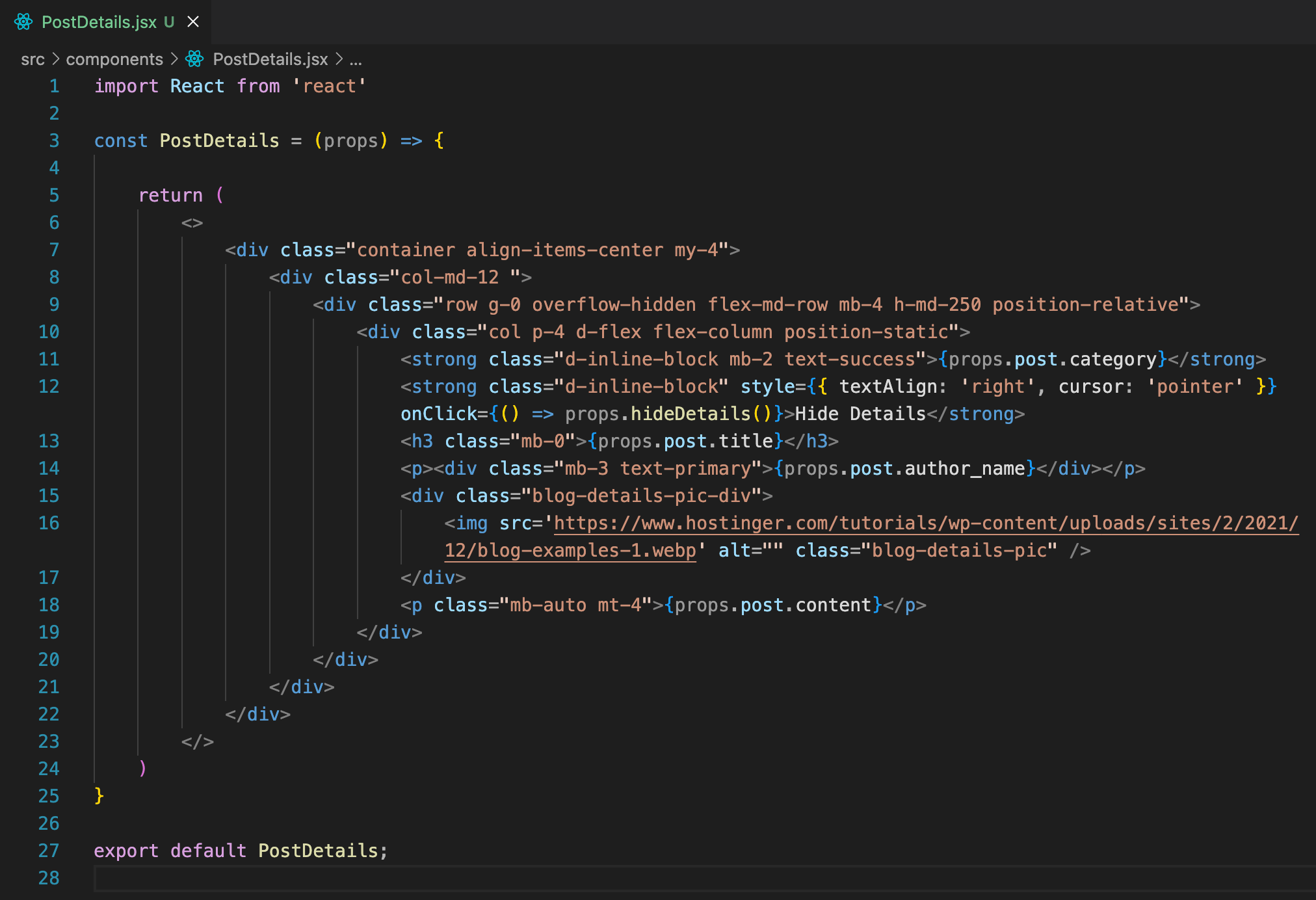
Now, write the following code for the “ListPost” component as shown in the image below.
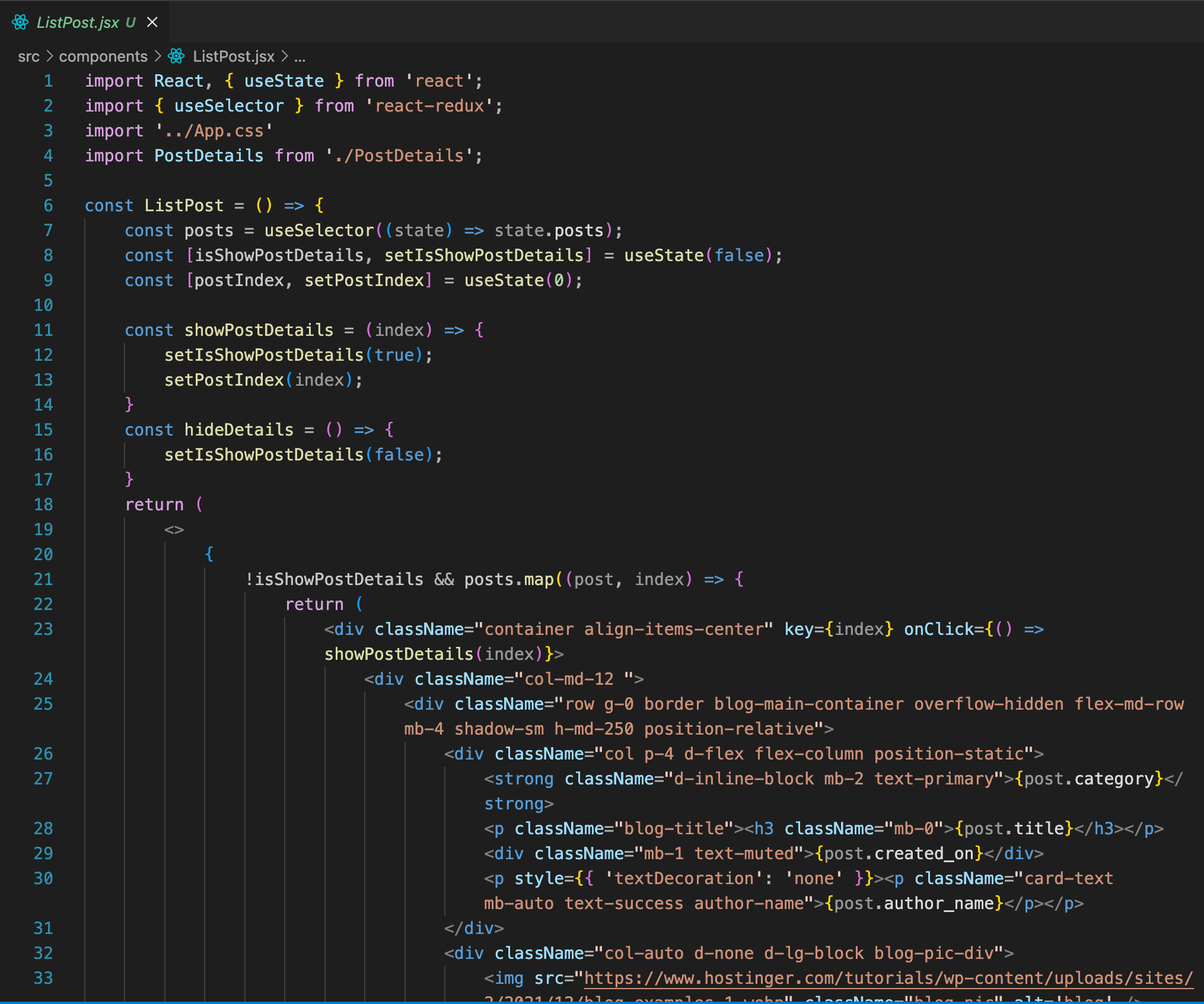
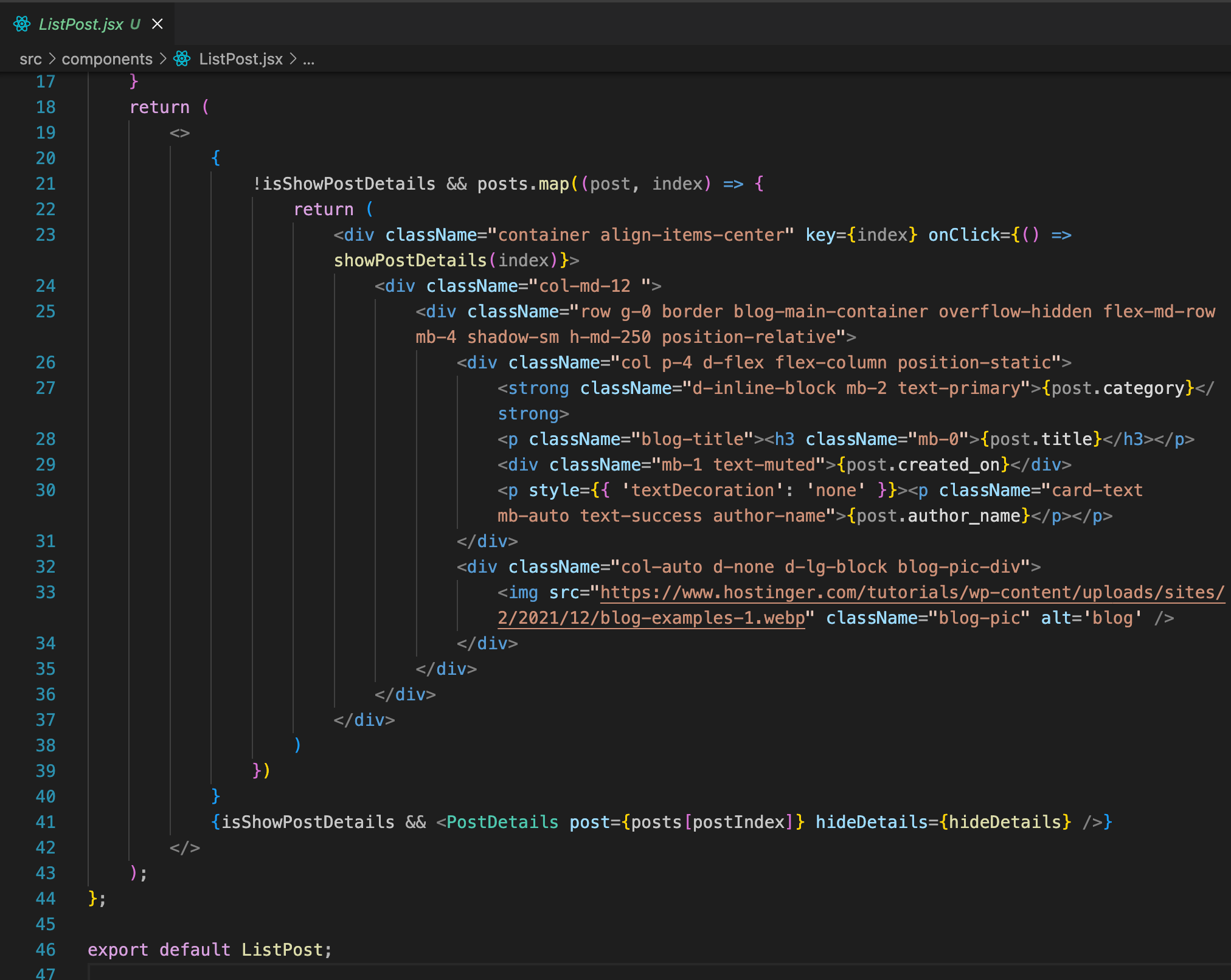
Add the “ListPost” component in the App.js file as shown in the image below.
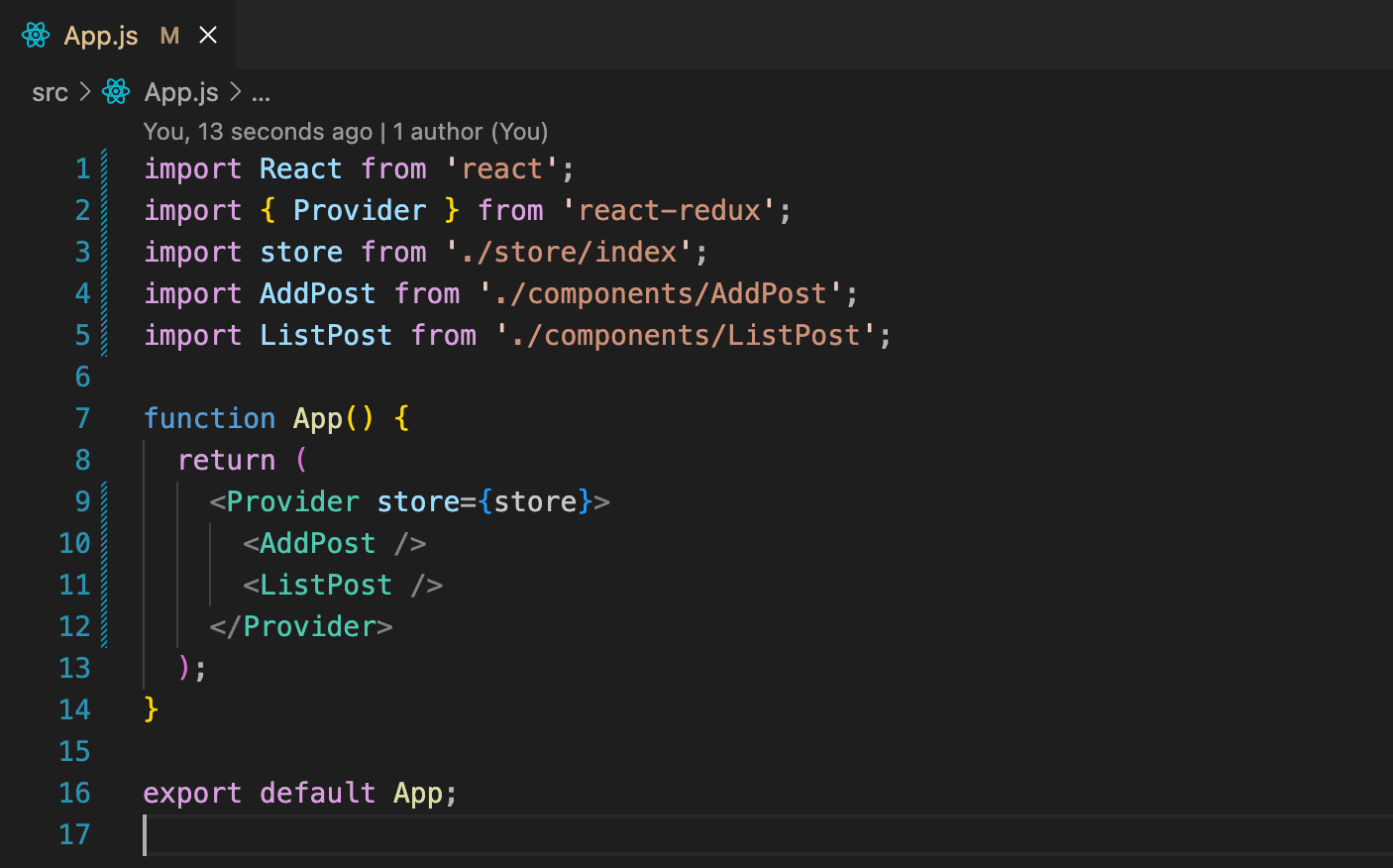
Open the browser and add a new post as shown in the image below.
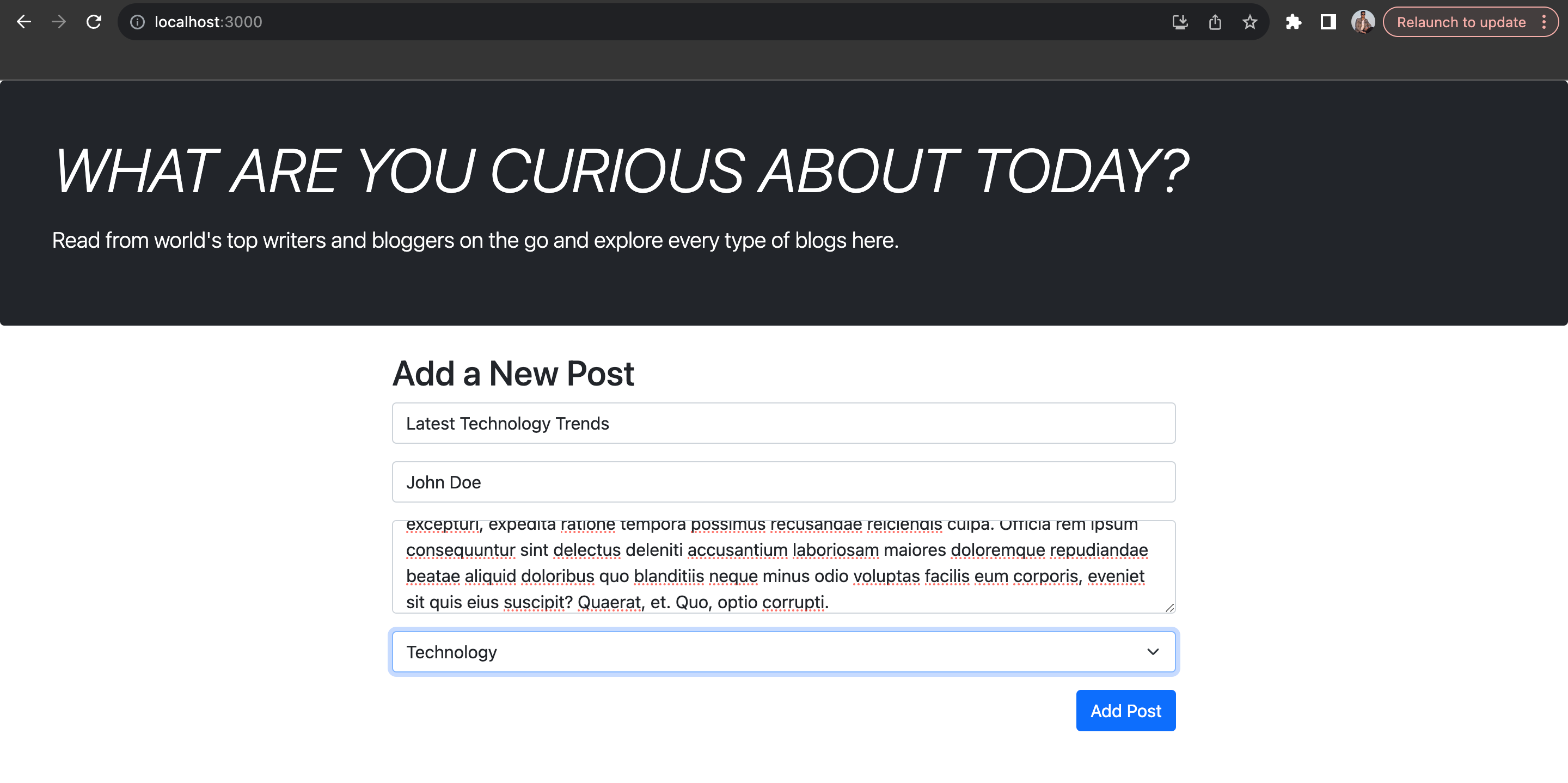
Click on “Add Post” button and it will automatically get added to the post list on the same page as shown in the image below.
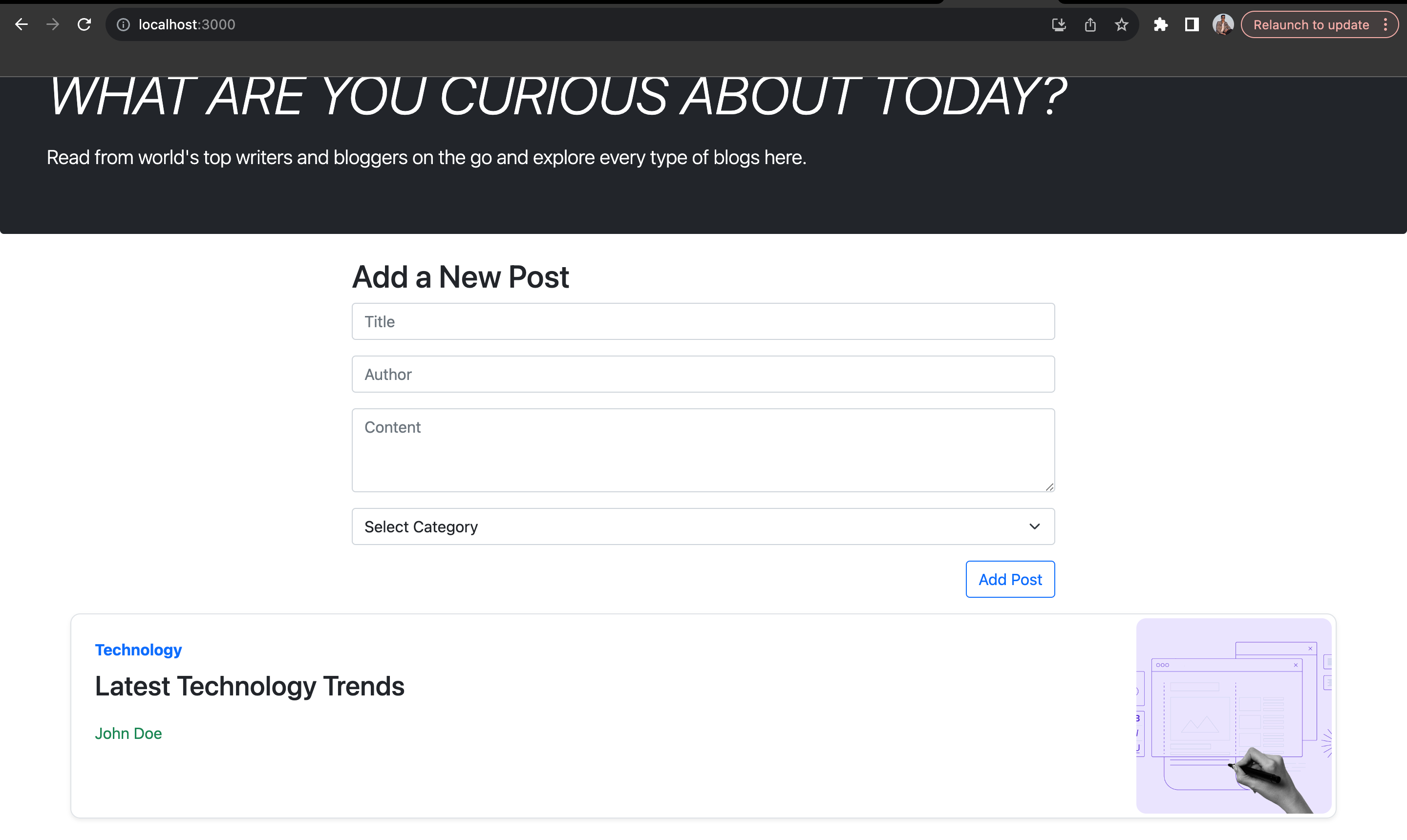
Click on the Post and you will be able to see the details of that post as shown in the image below.
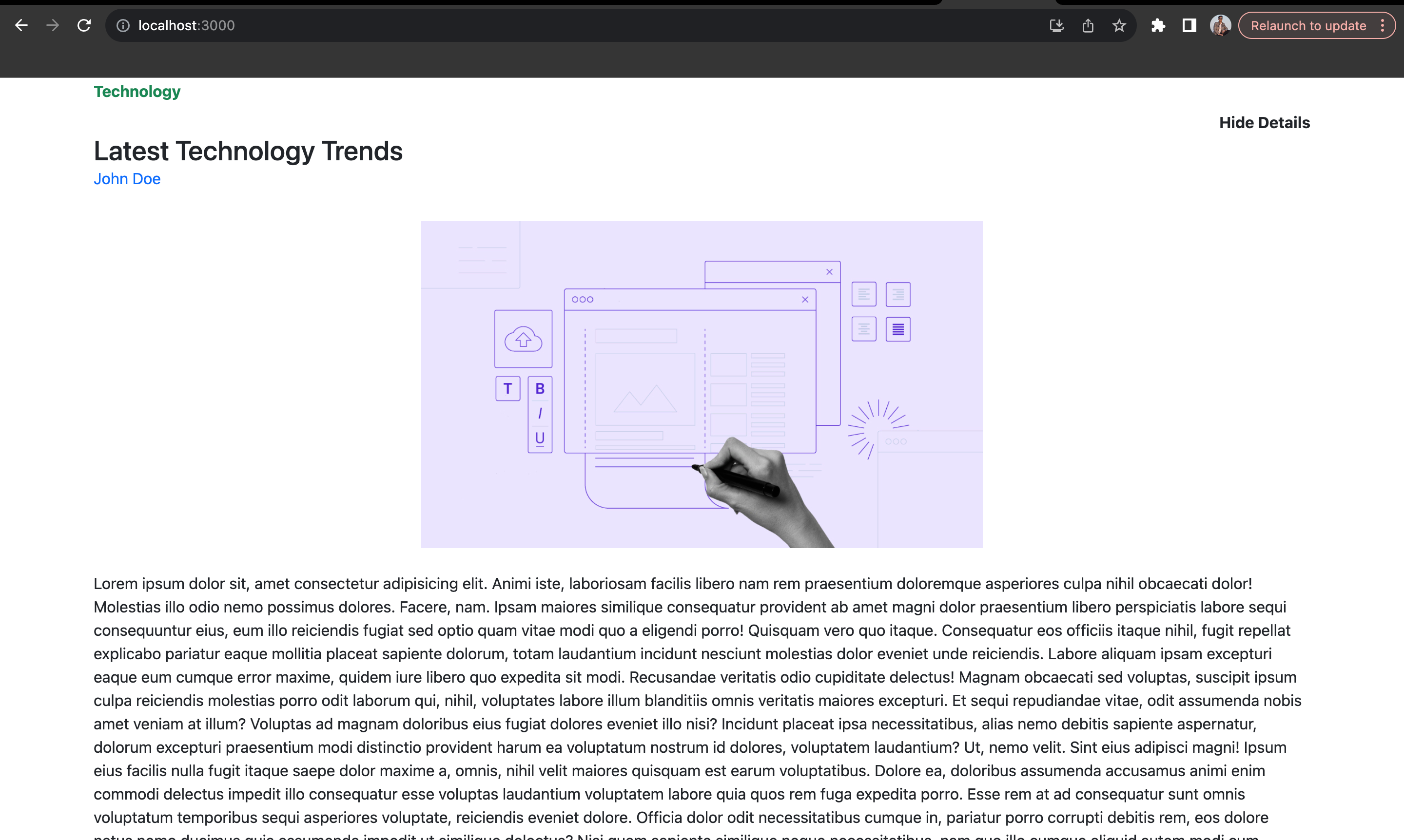
You can click on the “Hide Details” button to hide the details and go to the previous page.
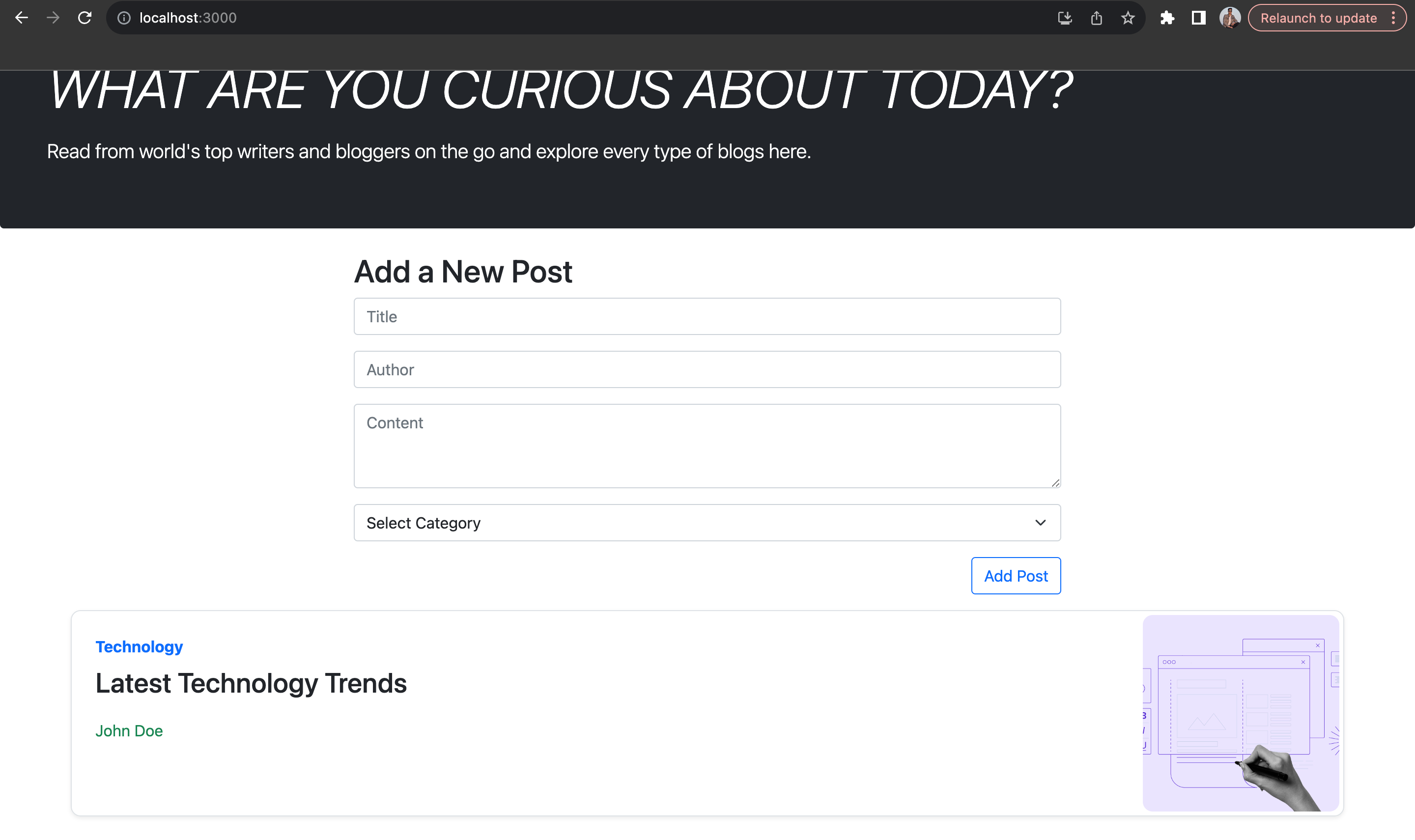
You can add multiple blogs and it will get appended in the post list automatically as shown in the image below.
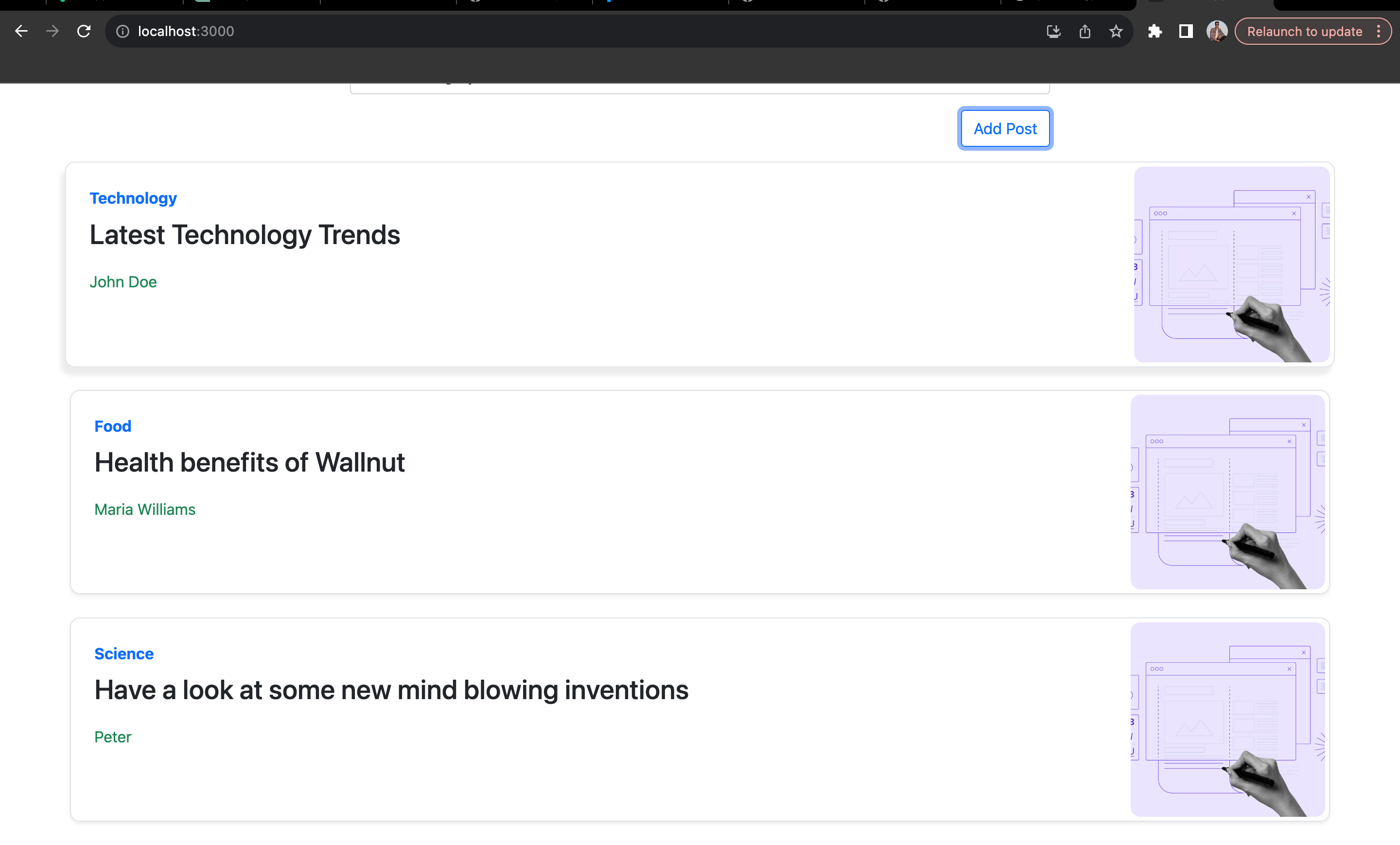
Conclusion
In conclusion, our journey through creating a React application with Redux for efficient state management involved several key steps. We initiated the process by creating a new directory on the local machine and setting up a React app in Visual Studio Code using the command “npx create-react-app blog-app.” The subsequent stages included the creation of essential components – ListPosts, AddPost, and PostDetails – along with the establishment of a Redux store. We installed the required libraries, defined reducers and actions, and integrated them into the application to manage state effectively.
The UI development phase encompassed creating the “AddPost” component with fields like title, author_name, category, and content. Adjustments were made to the “App.js” file, Bootstrap CDN was introduced, and styling was applied using provided CSS code in “App.css.” Further steps involved coding the “PostDetails” and “ListPost” components and seamlessly integrating them into the application.
Throughout the process, testing was conducted by adding new posts, viewing details, and navigating through a dynamically updated post list. The end result is a fully functional React application with Redux, showcasing effective state management and a dynamic user interface. This hands-on experience provided valuable insights into building versatile React applications, and we hope you’ve gained a deeper understanding of the implementation details discussed in the blog. We will come up with more such use cases in our upcoming blogs.
Meanwhile…
If you are an aspiring React developer and want to explore more about the above topics, here are a few of our blogs for your reference:
- How to Manage State in a React Application Using Redux?
- Supercharge Your React App with Real-Time GraphQL Subscriptions & Apollo Client
- How to upload large files (1GB and beyond) to AWS S3 using NestJS (backend) and ReactJS (frontend)?
- How to build and deploy dApps using Solidity Smart contract, WEB3.JS & React.JS?
- How to Build a Hybrid App using React Native?
- Angular vs React: Which one to choose and when?
Stay tuned to get all the updates about our upcoming blogs on the cloud and the latest technologies.
Keep Exploring -> Keep Learning -> Keep Mastering
At Workfall, we strive to provide the best tech and pay opportunities to kickass coders around the world. If you’re looking to work with global clients, build cutting-edge products, and make big bucks doing so, give it a shot at workfall.com/partner today!