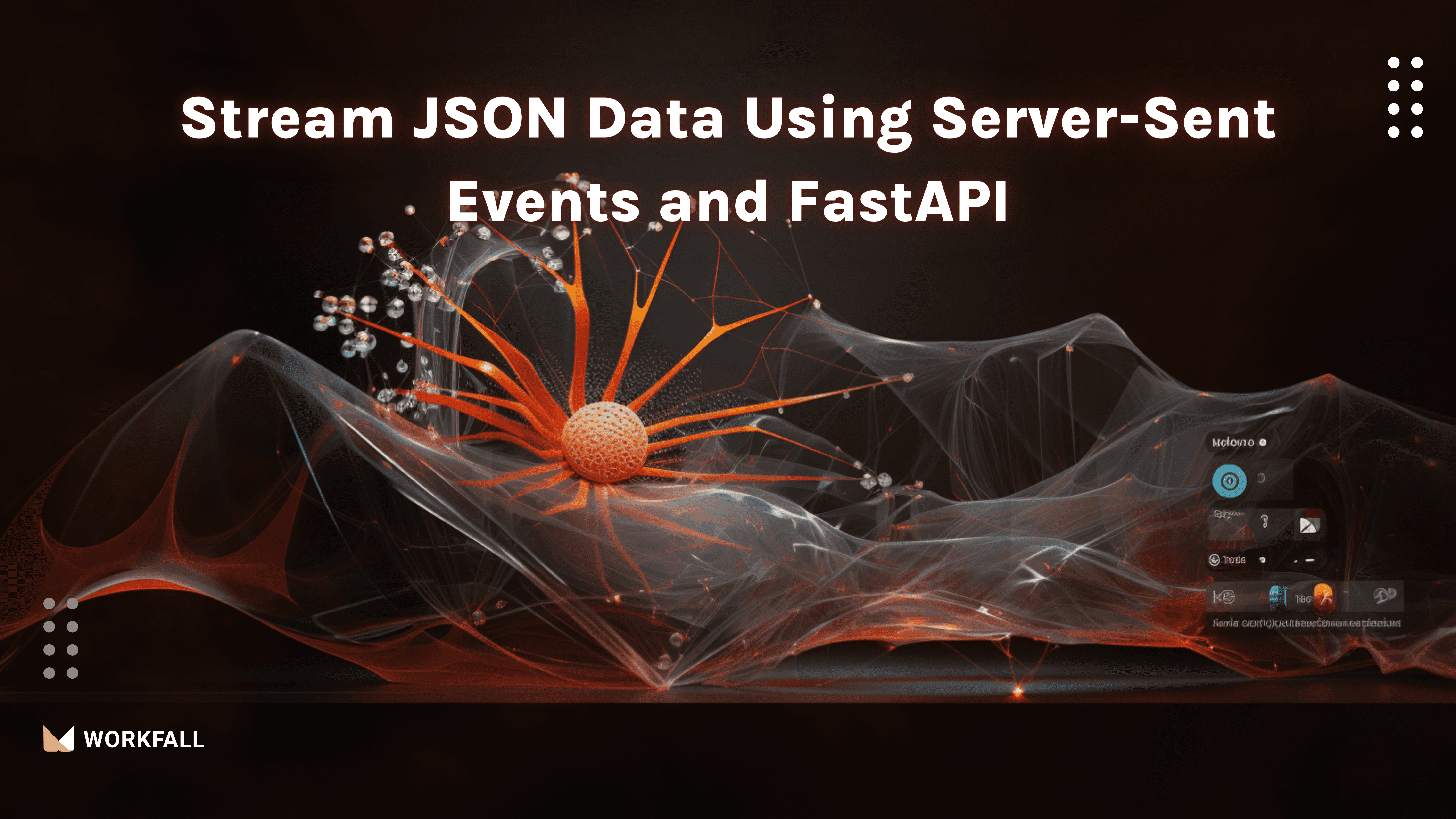
In this blog, we will cover:
- What are Server-Sent Events?
- Why Stream Data Using Server-Sent Events (SSE)?
- What is FastAPI?
- Hands-On
- Conclusion
What are Server-Sent Events?
Server-Sent Events (SSE) is a simple and efficient technology for sending real-time updates from the server to the web browser over a single HTTP connection.
Unlike other real-time communication methods that involve complex setups or polling, SSE relies on a unidirectional flow of data, where the server pushes events as text-based messages to the client. These events can carry information like notifications, updates, or alerts. SSE is standardized in HTML5 and is widely supported by modern browsers.
It’s particularly useful for scenarios where the client needs timely updates from the server without resorting to frequent requests, enhancing web applications’ responsiveness and efficiency.
Server-Sent Events (SSE) Architecture
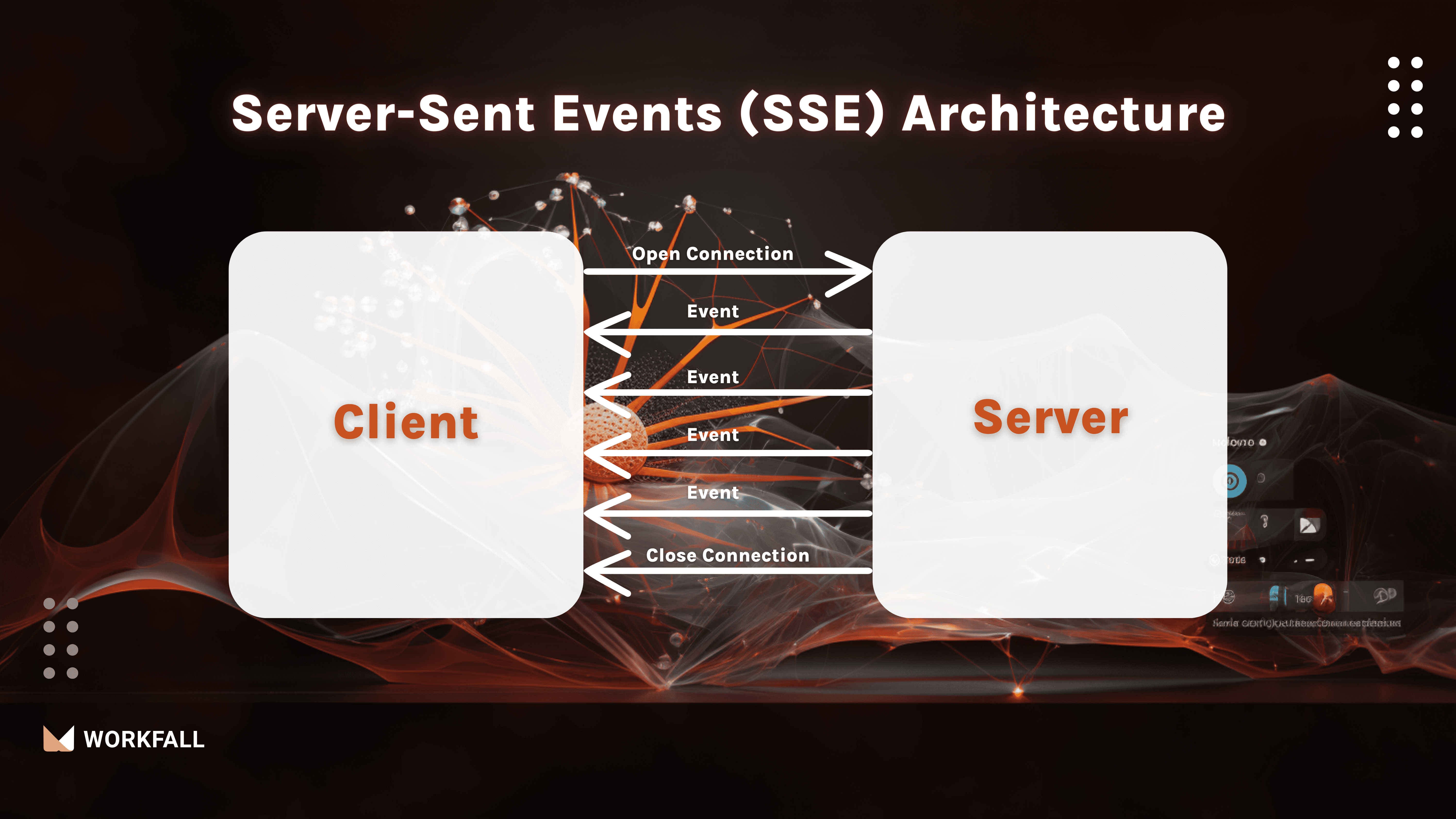
Why Stream Data Using Server-Sent Events (SSE)?
Streaming data using Server-Sent Events (SSE) addresses the need for efficient and real-time communication between web servers and clients.
Traditional web communication relies on the client repeatedly polling the server for updates, which can lead to unnecessary network traffic, increased server load, and delays in receiving new information.
SSE offers a more elegant solution by establishing a persistent and single HTTP connection between the client and server.
One significant advantage of SSE is its simplicity of implementation. Unlike other real-time technologies that might require more complex setups like WebSockets or third-party libraries, SSE is based on standard HTTP and can be easily integrated into existing web applications.
This makes it accessible to a wider range of developers and projects.
What is FastAPI?
FastAPI is a modern, high-performance web framework for creating Python APIs using standard type hints. It has the following main features:
- Fast to run: Its performance is comparable to NodeJS and Go, owing to Starlette and pydantic.
- Fast to code: It enables considerable speed increases in development.
- Reduced number of bugs: It lowers the probability of human-caused errors.
- Intuitive: It provides excellent editor assistance, with completion everywhere and reduced debugging time.
- Straightforward: It is intended to be simple to use and learn so that you may spend less time reading documentation.
- Short: It reduces code duplication.
- Robust: It delivers production-ready code with interactive documentation.
- Standards-based: It is based on the open API standards OpenAPI and JSON Schema.
The framework is intended to improve your development experience by allowing you to write simple code to create production-ready APIs that follow best practices by default.
Hands-On
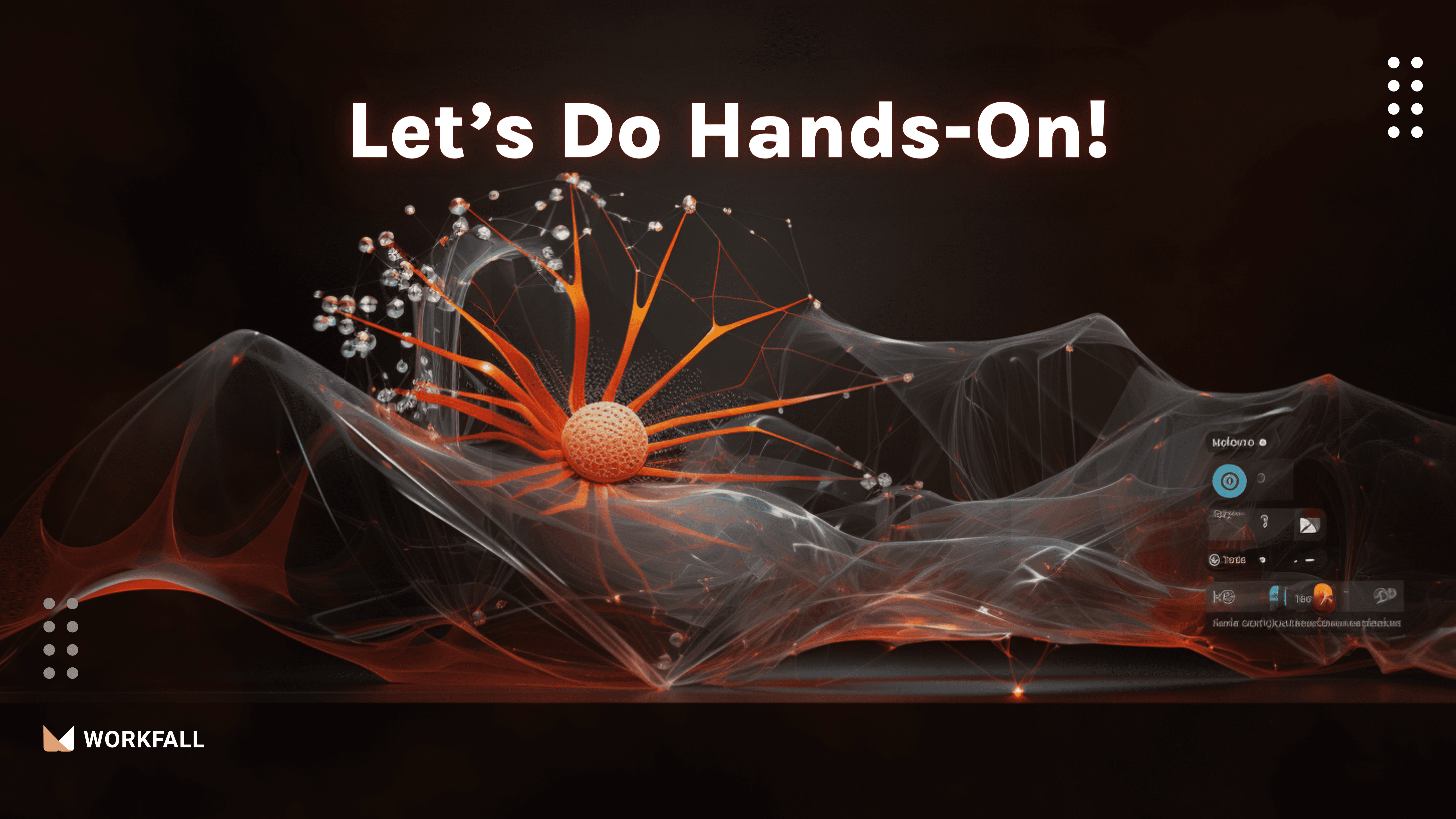
Required installations:
To perform the demo, you require the following installations:
- aiohttp: It is a Python library for asynchronous HTTP client/server communication, built on top of asyncio.
- FastAPI: FastAPI is a modern, high-performance web framework for building APIs with Python.
- UVicorn: UVicorn is a fast ASGI server for running Python web applications, designed for high performance and compatibility with asynchronous code.
- requests: requests is a library used for making HTTP requests in Python with a user-friendly interface.
In this hands-on, we’ll learn to use Server-Sent Events to smoothly stream JSON data using FastAPI. We’ll start by making a new project in PyCharm IDE and installing the required libraries. Our coding journey will kick off with setting up FastAPI in the project.
Then, we’ll dive into the server-side code. Initially, we’ll cover basic text streaming and then progress to streaming JSON events.
Switching gears to the client side, we’ll work on the logic there. Along the way, we’ll thoroughly test our API multiple times to ensure everything functions as expected. Our end goal is to have a fully functional solution that can stream JSON events in real-time.
This technique is super handy when we need to make lots of API calls quickly, which can be costly for the app.
By harnessing the power of Server-Sent Events, we’ll find out how to smartly handle this situation, saving resources and improving efficiency.
By the end, we’ll have a grasp of an effective method to keep data flowing smoothly in our applications without unnecessary overheads.
Open PyCharm CE on your desktop and click on ‘New Project’.
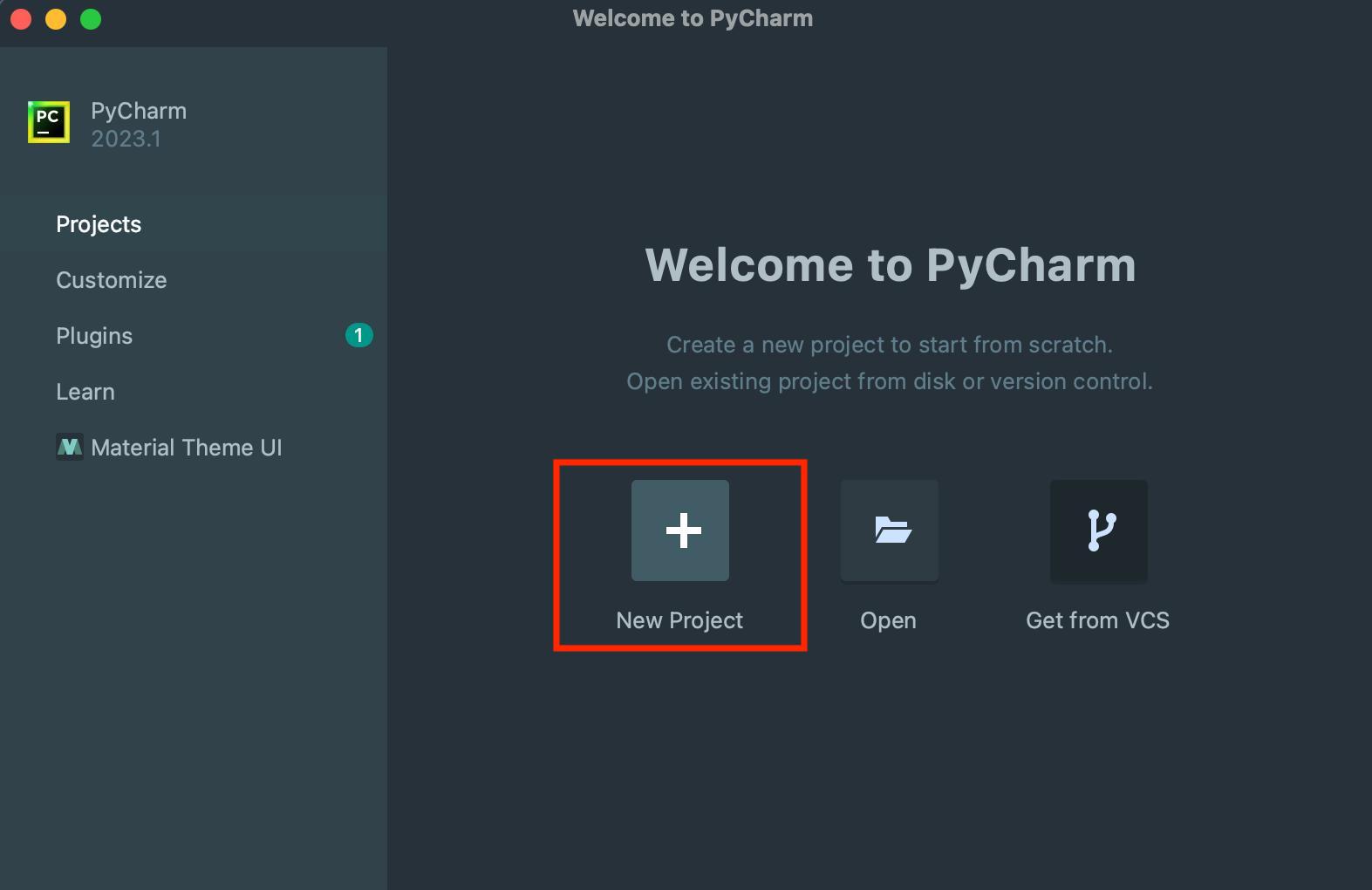
Name your project and choose the path to save your project and then click on ‘create’.
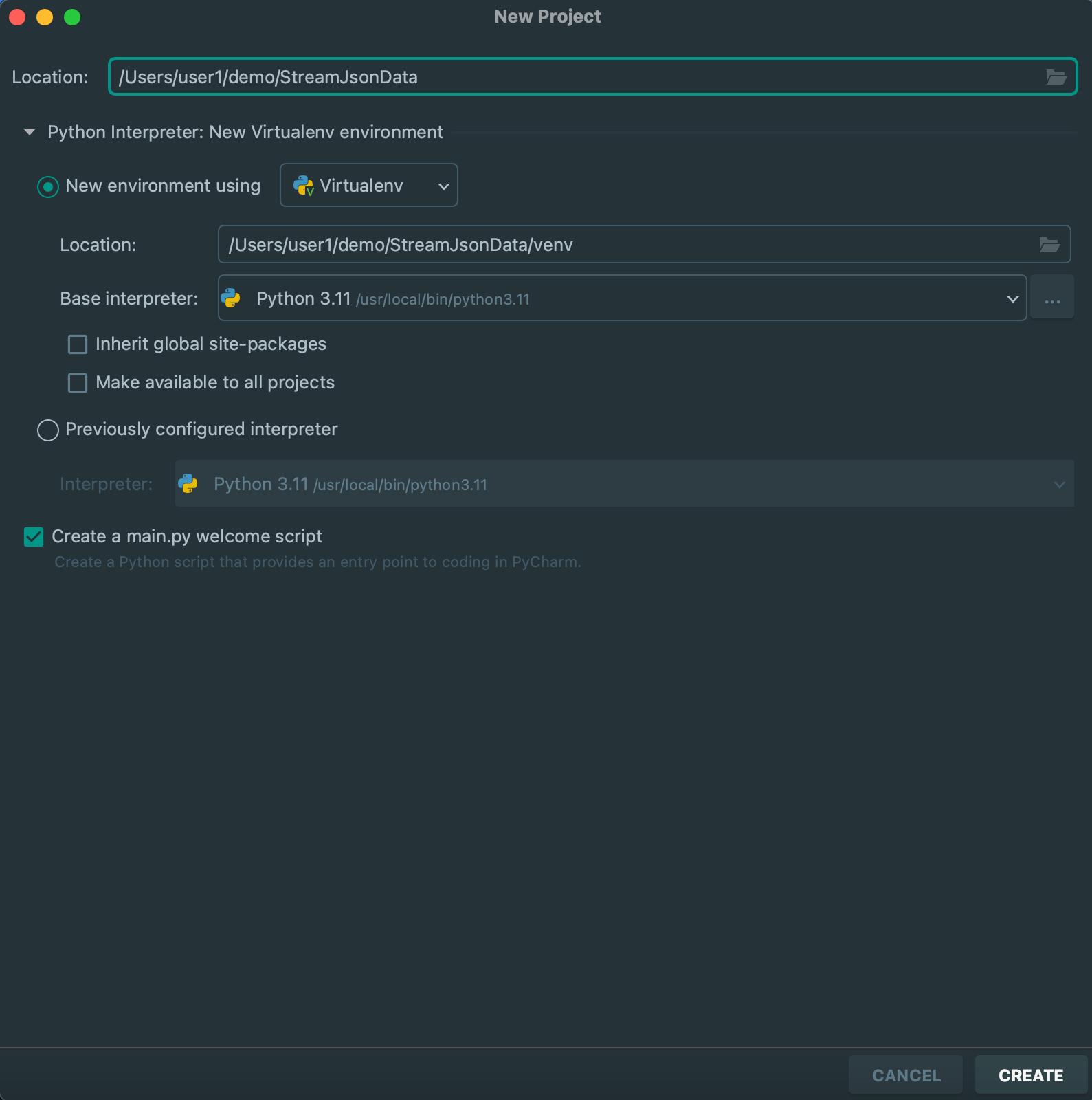
Remove all the auto-generated code from the main.py file.
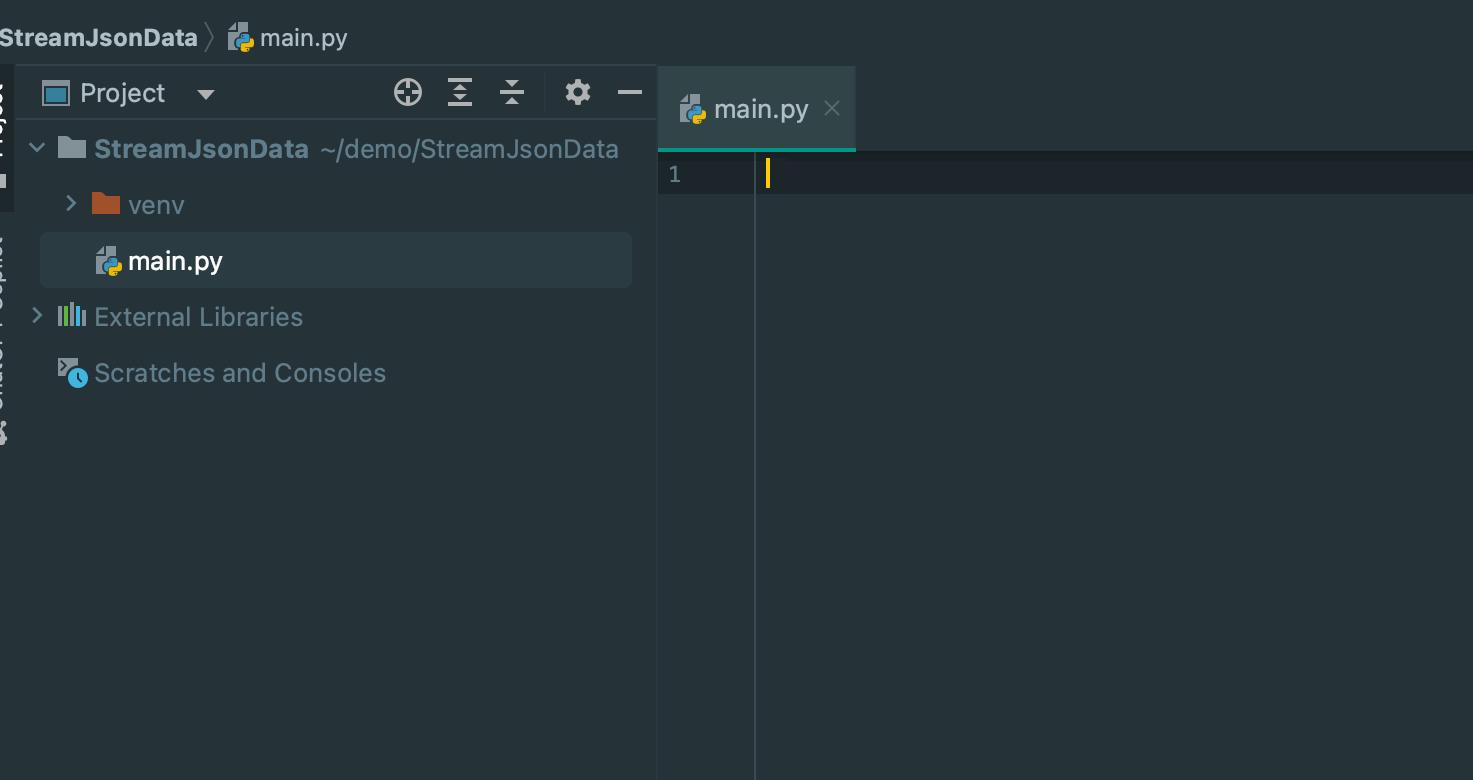
Open the PyCharm terminal and run the command as shown in the image below to install the FastAPI framework.

On successful installation, you will see the screen as shown in the image below.
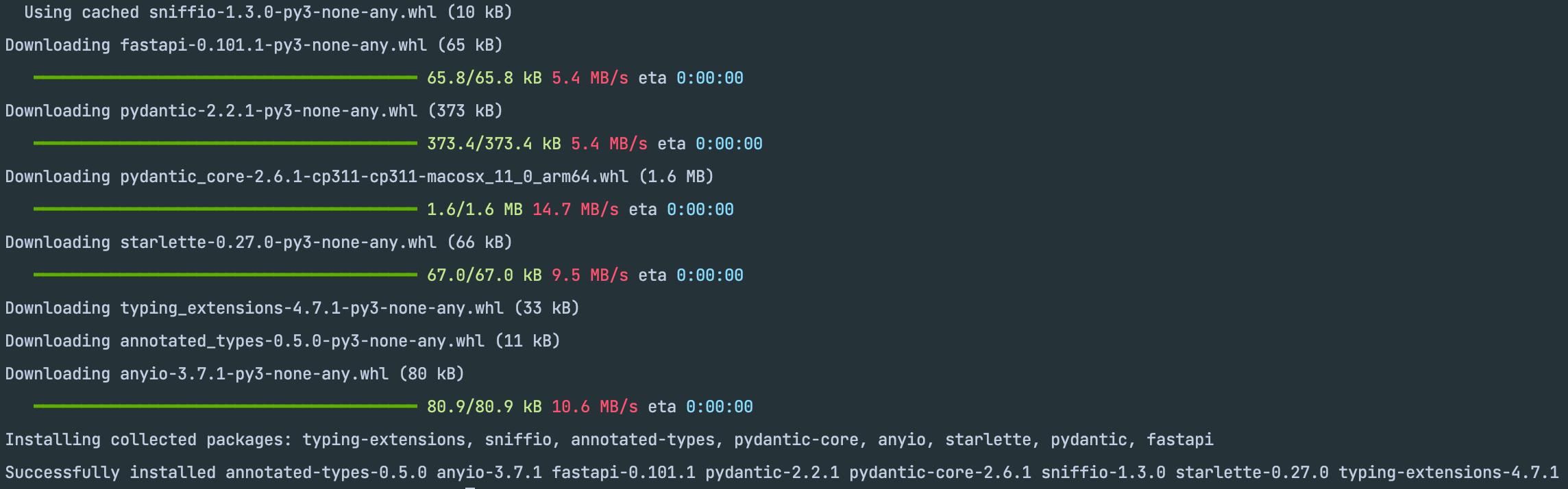
Run the command as shown in the image below to install the UVicorn server.

On successful installation, you will see the screen as shown in the image below.
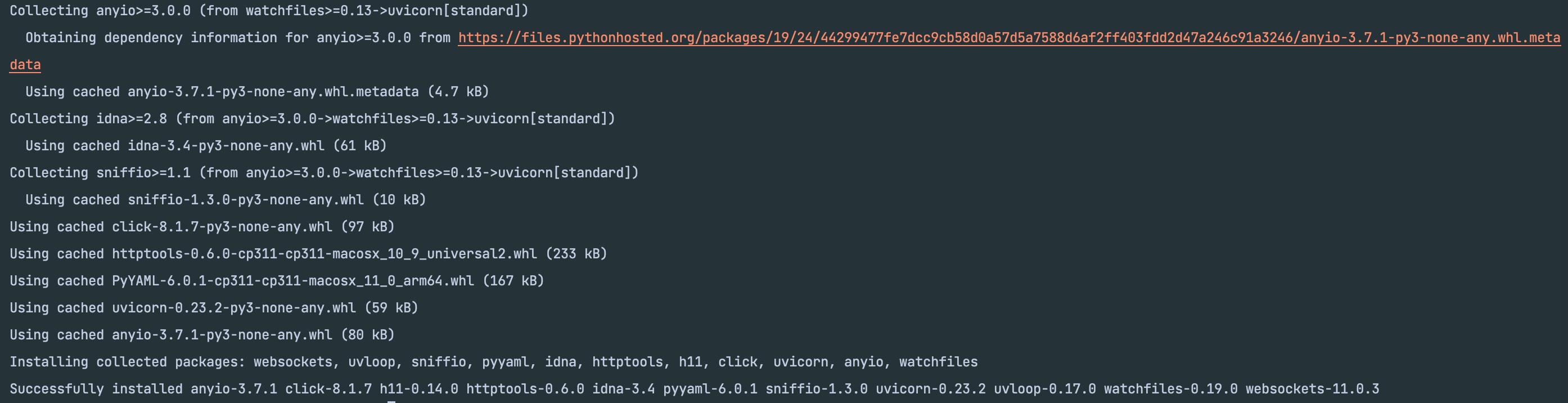
Run the command as shown in the image below to install the aiohttp library.

On successful installation, you will see the screen as shown in the image below.

Let’s create a demonstration API route and perform testing with FastAPI.
Import the FastAPI class as shown in the image provided.

Instantiate the FastAPI application using the FastAPI class as shown in the provided image.

Create a root route at ‘/’ that provides a placeholder message in response.
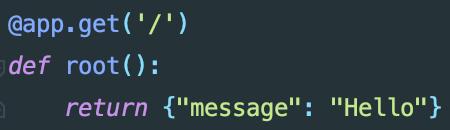
The final code will look as shown in the image below.
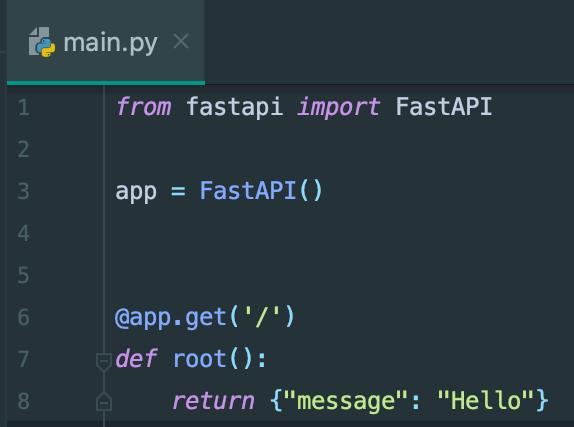
Initiate the server using the command demonstrated in the provided image.

After running the command the server will get started and you will see a screen as shown in the image below.
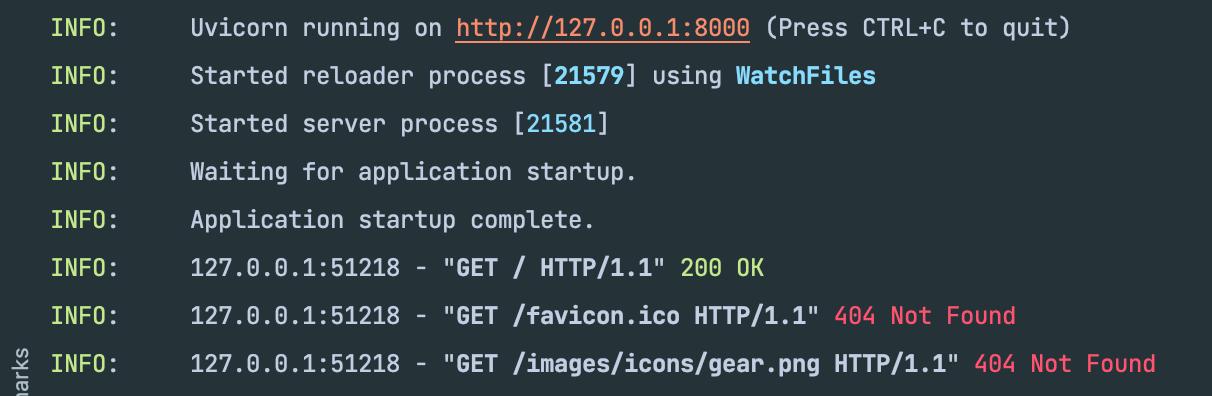
Click on the link shown in the terminal to see the message that we returned from our code.
If everything goes well, you will be able to see a message on your browser as shown in the image below.
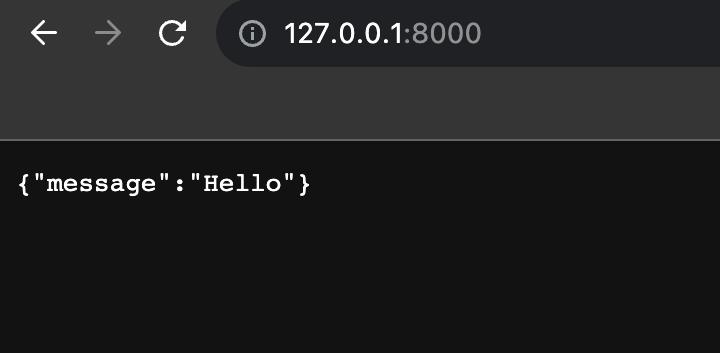
Let’s begin by exploring the process of streaming plain text before we dive into streaming JSON data.
FastAPI framework provides a built-in class called StreamingResponse, which allows us to retrieve data in segmented portions or chunks.
Let’s change our existing code to stream basic text message.
Import the StreamingResponse library and asyncio library as shown in the image below.

Create a simulated generator function that mimics the streaming process.
This function will produce a sequence of plain text strings within a loop that iterates 10 times. Between each iteration, the program will pause for 1 second before proceeding to the next iteration.
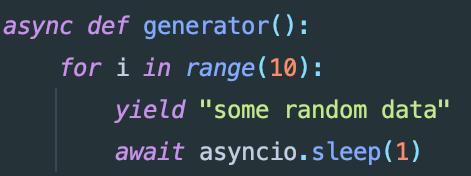
Let’s invoke our generator function and provide it as an argument to the constructor of StreamingResponse.
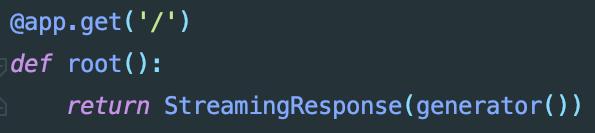
Now, let’s write the client-side logic of our application to seamlessly fetch the data as a stream from the server
Create a new file and name it client.py.
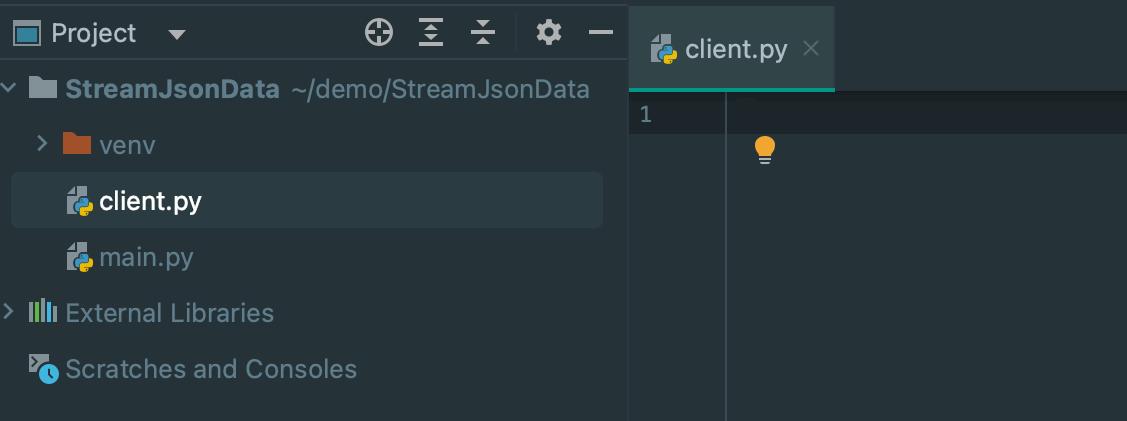
Install the requests library using the command shown in the image below.

Import the requests library as well as the datetime library as shown in the image below.
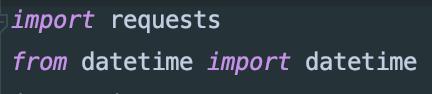
Store the url of our server in a variable as shown in the image below.

This will transfer information in one go over an HTTP connection from the server to the client. We can easily retrieve the data using Python’s “requests” library, as shown in the image below.

We’re taking in 16 bytes of data at a time from the stream. Sometimes, cutting off the data partway doesn’t cause issues.
But usually, we’ll be getting organized data that we’ll want to put back together, and for those situations, we need to know where each piece of data starts and finishes.
The final code will look like as shown in the image below
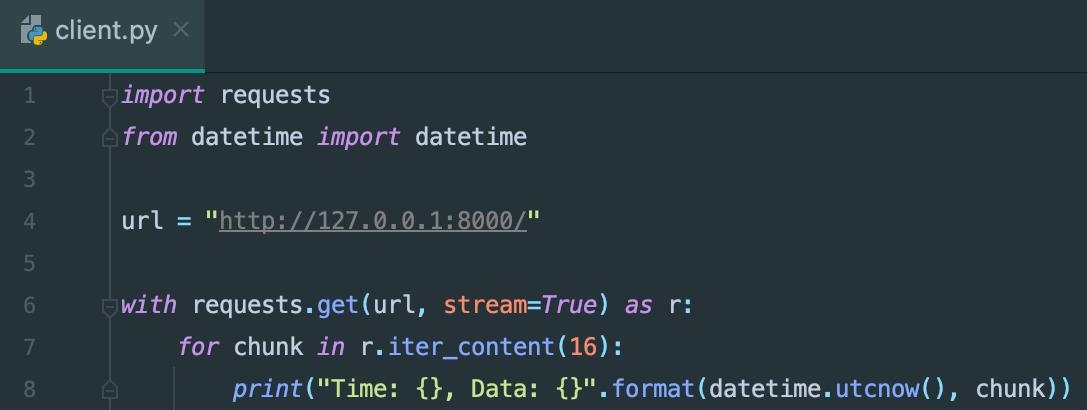
Run the code using the command shown in the image below.

Once the program runs successfully, we will get our output as a nice stream of data.
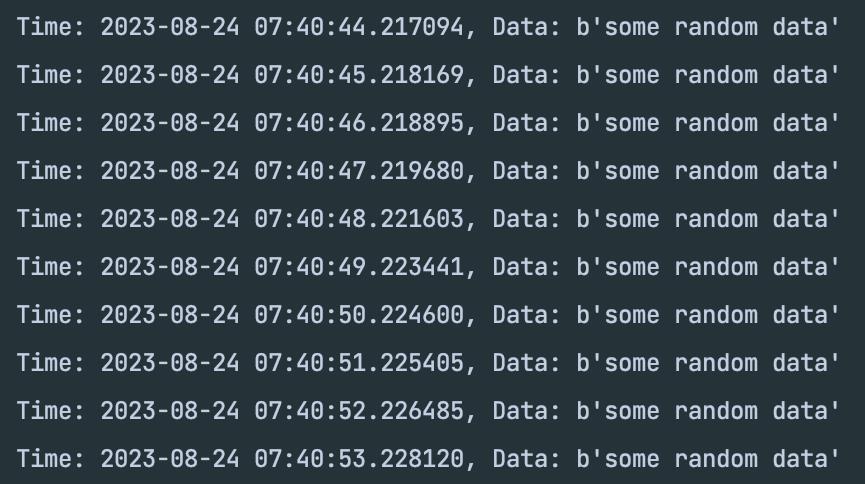
Next, Let’s move to Streaming JSON Events.
Our objective is to stream the JSON data something like this:
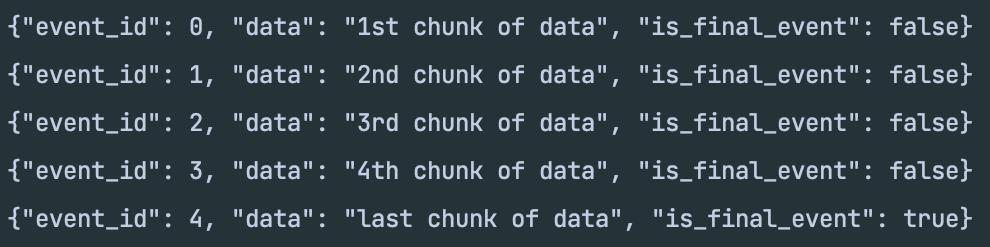
Ideally, the client should be able to read the stream like a collection. They can be confident that each time they iterate through it, they’ll have a complete JSON object. This makes it easy for the client to understand and work with.
To make it possible, we need to adjust our server to stream JSON objects instead of plain text.
Adjust the code in the main.py file as shown in the image below.
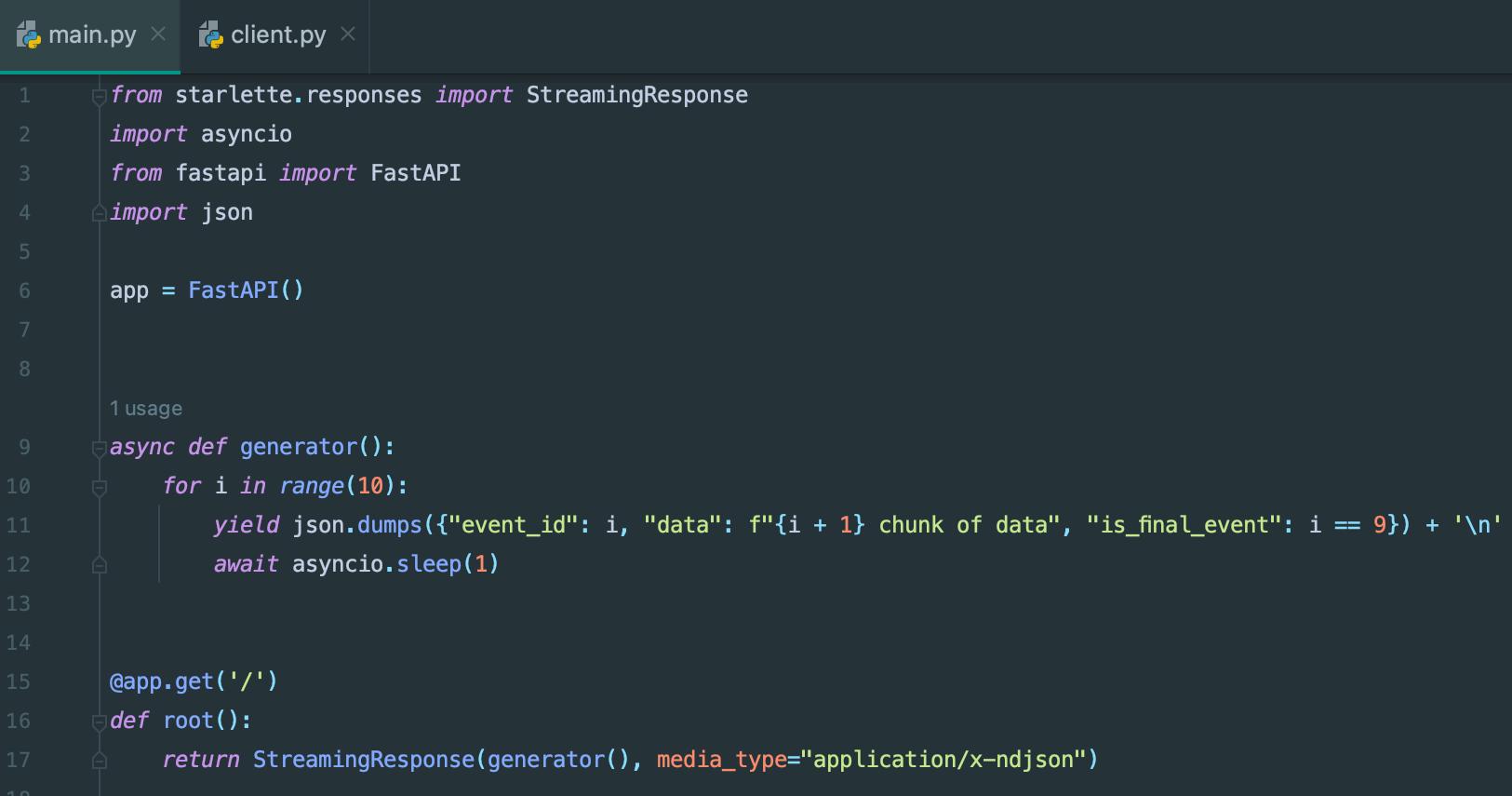
Every event will be written as a JSON object in a row, and each event will be separated by a new line. We’re also using the media type “application/x-ndjson,” which just means that each JSON object is on its own line. We looked into it, and it seems like this is the right type to use. This should make things easier for you.
With these changes, the client can use the “readline()” function to easily read a stream of lines. This function will provide basic units of data in the form of raw bytes.
These bytes can then be converted into a readable JSON format. By adding a few more convenient features, we can achieve the user-friendly interface we wanted. Look at the example provided in the image below.
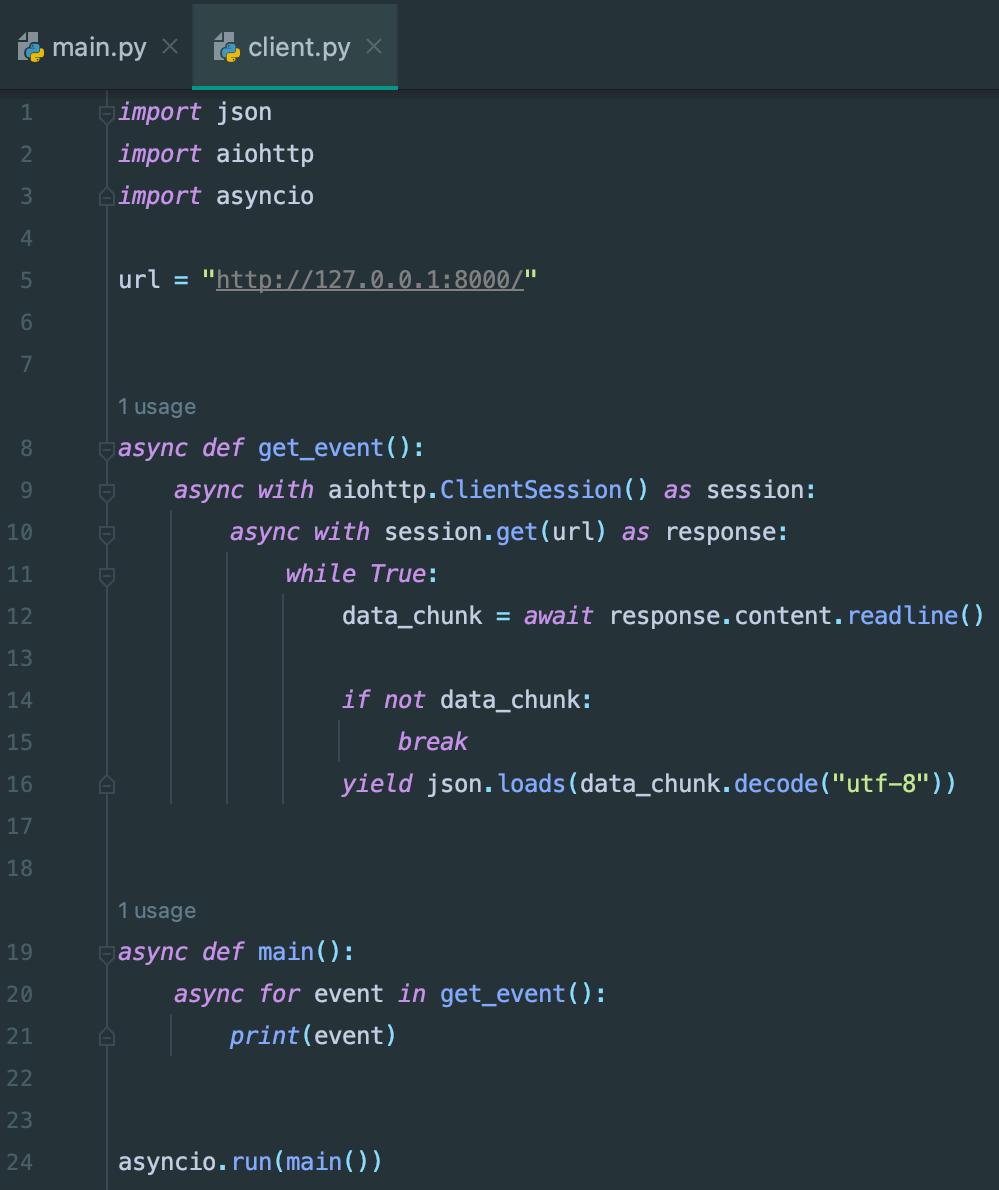
Let’s run the program using the command shown in the image below.

After running the command, we can observe a nice flow of JSON events being created as shown in the image below.
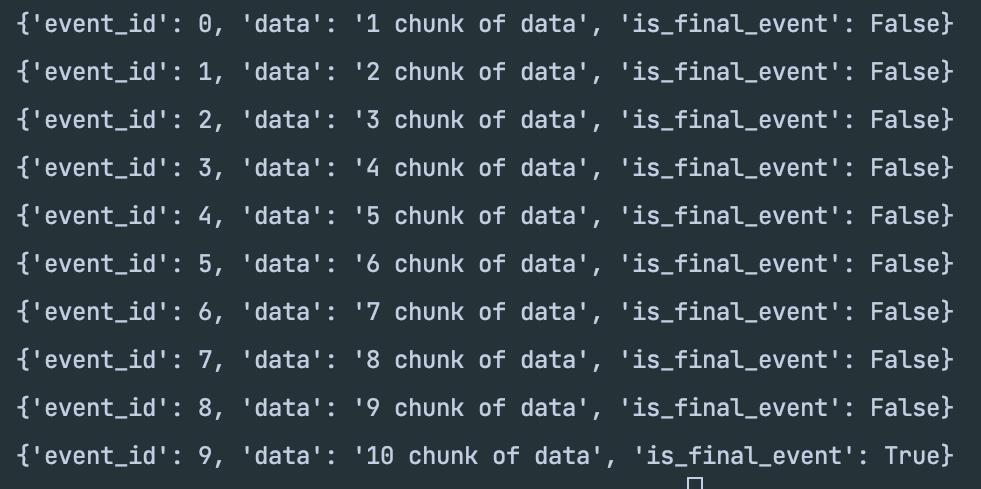
This code can be written in a synchronous way as well. Refer to the below example.
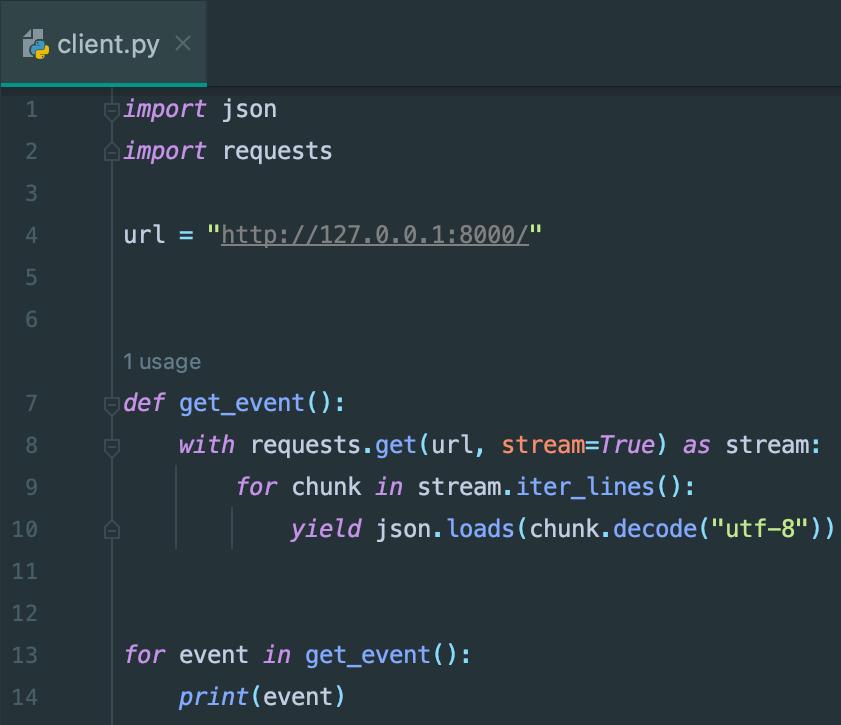
Conclusion
In this hands-on project, we learned about using Server-Sent Events to smoothly stream JSON data using FastAPI. We started by making a new project using PyCharm IDE and putting in the necessary stuff we need. Then, we got FastAPI set up in our project.
After that, we did some coding on the server side. First, we practiced sending simple text, and later, we moved on to sending JSON events.
On the other side of things, we worked on the part that gets the information from the server i.e., the client. While doing this, we made sure to test our work to make sure it was doing what we wanted.
Our big goal was to create something that could send JSON information in real-time. We successfully achieved this using both synchronous and asynchronous ways.
This method becomes really useful when we need to do a lot of things quickly without spending too much. By using Server-Sent Events, we found a clever way to handle this and make things better.
At the end of it all, we figured out a great method to keep information moving smoothly in our apps without making things complicated.
We will come up with more such use cases in our upcoming blogs.
Meanwhile…
If you are an aspiring Python developer and want to explore more about the above topics, here are a few of our blogs for your reference:
- How to Create an Amazon Price Tracker Service Using Python?
- How to Read and Write In Google Spreadsheet Using Python and Sheety API?
- How to Execute Linux Commands in Python?
Stay tuned to get all the updates about our upcoming blogs on the cloud and the latest technologies.
Keep Exploring -> Keep Learning -> Keep Mastering
At Workfall, we strive to provide the best tech and pay opportunities to kickass coders around the world. If you’re looking to work with global clients, build cutting-edge products, and make big bucks doing so, give it a shot at workfall.com/partner today!